In this post I am going to explain URL routing in greater details. This post will contain basic of URL routing and will explain how we can do URL routing in fewer lines of code.
Now as mapping code has been done let’s right code for customer.aspx page. I have have following code in page_load event of customer.aspx
Here in above code you can see that I am getting value from page route data and then just printing it. In real world it will fetch customer data from database and show customer details on page.
Now let’s run that application. It will print a details of customer as I have passed Id in URL suppose you pass 1 as id in URL then it will look like following.
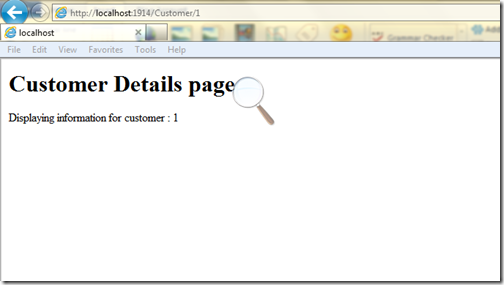
Now if you put 2 in url it will print information about customer 2.
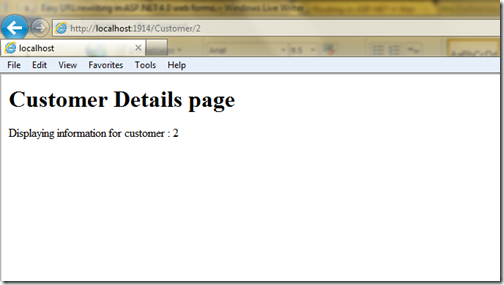
That’s it. So we have enabled URL routing in asp.net in fewer lines of code. In next post I am going to explain redirection with URL routing.
Hope you like this post. Stay tuned for more.. Till then Happy programming
Why we need URL routing ?
Let’s consider a simpler scenario we want to display a customer details on a ASP.NET Page so how our page will know that for which customer we need to display details? The simplest way of doing is to use query string we will pass a customer id which uniquely identifies customer in query string. So our url will look like this.
Customer.aspx?Id=1
This will work but the problem with above URL is that its not user friendly and search engine friendly. who is going to remember that what query string parameter I am going to pass and why we need that parameter. Also when search engine will crawl this site it will going to read this URL blindly as this url is not informative because it query string is not readable for search engine crawlers. So your search engine will be ranked lower as this URL is not readable to search engine crawlers.Now when do a URL routing our URL will be cleaner shorter and simpler like this.
Customers/Id/1/
Here anybody in world can understand it talking about customer and this page will used to show customer details.Even search engine crawler will also know that you are talking about customers. That is why we need URL routing.
URL routing and ASP.NET
In earlier versions of ASP.NET we have to write lots of code for URL routing but Now with ASP.NET 4.0 you can easily route in fewer lines of code.
So let’s start a Demo where I will demonstrate you how we can easily route URLs. So let’s first create a ASP. NET web form application via File->New->Project and a dialog box will open just like below and then created a empty project called URL rewriting.
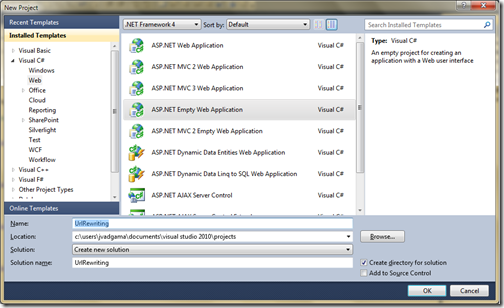
After creating a project I have added global.asax – where we are going to write url mapping logic and then I have added an asp.net page called which will display customer information just like below.
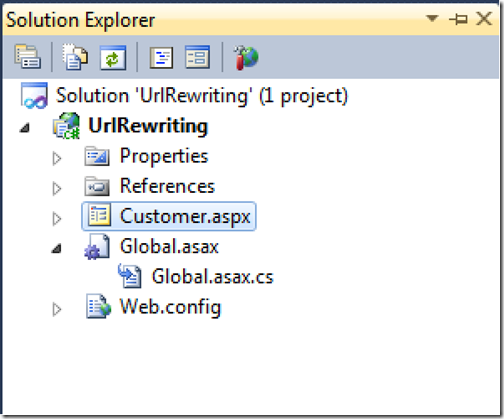
So everything is now ready let’s start writing code. First thing we need to do is to define routes. Route will map a URL to physical page.First I will create static function called Register route which map route to particular file and then I am going to call this from application_start event of global.asax. Following is code for that.
So let’s start a Demo where I will demonstrate you how we can easily route URLs. So let’s first create a ASP. NET web form application via File->New->Project and a dialog box will open just like below and then created a empty project called URL rewriting.
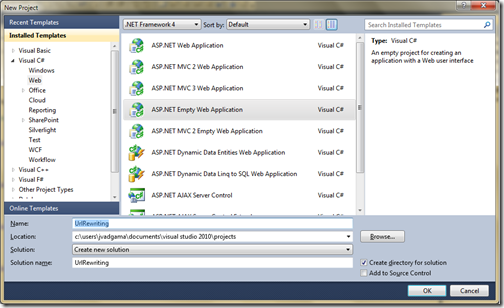
After creating a project I have added global.asax – where we are going to write url mapping logic and then I have added an asp.net page called which will display customer information just like below.
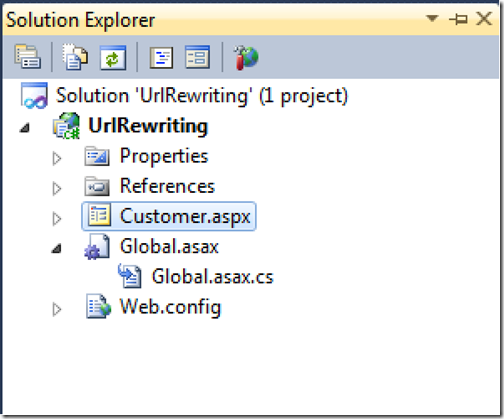
So everything is now ready let’s start writing code. First thing we need to do is to define routes. Route will map a URL to physical page.First I will create static function called Register route which map route to particular file and then I am going to call this from application_start event of global.asax. Following is code for that.
using System; using System.Web.Routing; namespace UrlRewriting { public class Global : System.Web.HttpApplication { protected void Application_Start(object sender, EventArgs e) { RegisterRoutes(RouteTable.Routes); } public static void RegisterRoutes(RouteCollection routeCollection) { routeCollection.MapPageRoute("RouteForCustomer", "Customer/{Id}", "~/Customer.aspx"); } } }
Now as mapping code has been done let’s right code for customer.aspx page. I have have following code in page_load event of customer.aspx
using System; namespace UrlRewriting { public partial class Customer : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { string id = Page.RouteData.Values["Id"].ToString(); Response.Write("<h1>Customer Details page</h1>"); Response.Write(string.Format("Displaying information for customer : {0}",id)); } } }
Here in above code you can see that I am getting value from page route data and then just printing it. In real world it will fetch customer data from database and show customer details on page.
Now let’s run that application. It will print a details of customer as I have passed Id in URL suppose you pass 1 as id in URL then it will look like following.
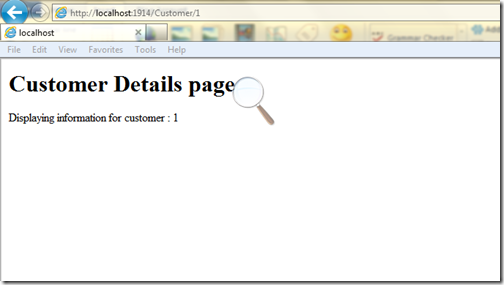
Now if you put 2 in url it will print information about customer 2.
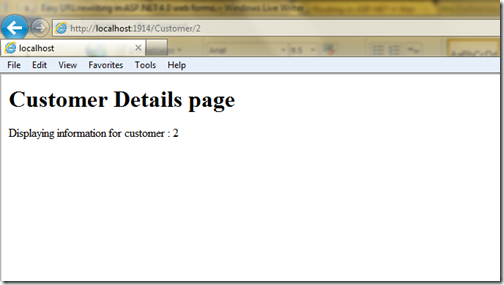
That’s it. So we have enabled URL routing in asp.net in fewer lines of code. In next post I am going to explain redirection with URL routing.
Hope you like this post. Stay tuned for more.. Till then Happy programming