This post may be pretty basic for many people. But I have been getting this request over and over so I thought it will be a good idea to write a blog post about it.
In this blog post, We are going to learn how we can create the cascading dropdown with ASP.NET Web forms and Entity framework. To create an application first we need to create web application like following.
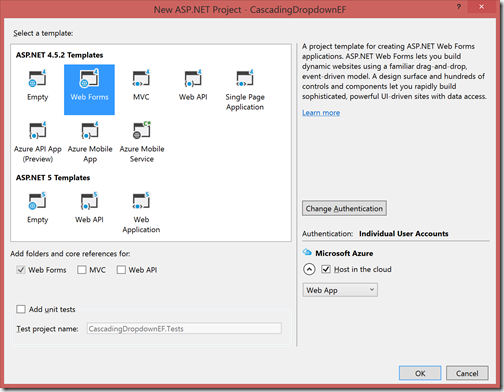
After creating a web application like following. It’s time to create model classes for our application. Here to illustrate the example, We are going to use Standard and Student models. Multiple students can be there in a standard. So we are going to have two dropdowns Standard and Student. Once you change select standard based on that student dropdown will be filled.
Here is code for Standard Student Model class:
Now we have our database layer or operation ready. it’s time to create a new Web Form which will have Standard and Student drop-down.

After creating Web Form I have written following HTML code in aspx file.
On the aspx.cs file i have written the following code.
Now everything is ready. I have inserted following data in Standard and Student table.

And student table like following.

Now when you run the application. It will look like following.
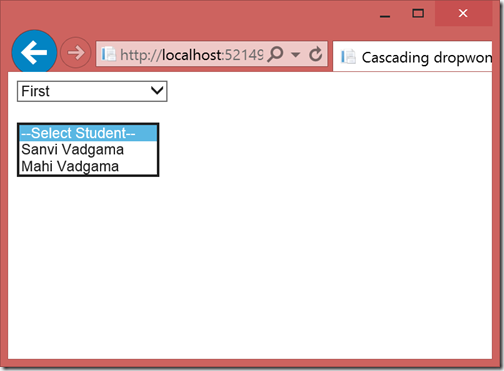
That’s it. Hope you like it. Stay tuned for more.
In this blog post, We are going to learn how we can create the cascading dropdown with ASP.NET Web forms and Entity framework. To create an application first we need to create web application like following.
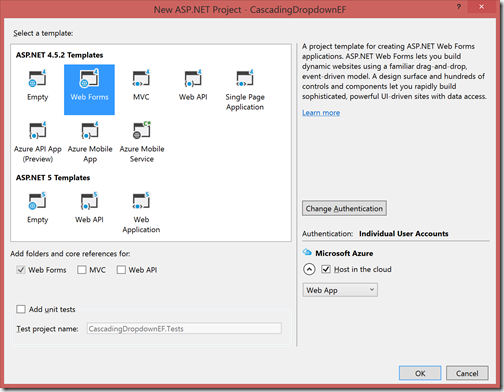
After creating a web application like following. It’s time to create model classes for our application. Here to illustrate the example, We are going to use Standard and Student models. Multiple students can be there in a standard. So we are going to have two dropdowns Standard and Student. Once you change select standard based on that student dropdown will be filled.
Here is code for Standard Student Model class:
using System.Collections.Generic; namespace CascadingDropdownEF.Models { public class Standard { public int StandardId { get; set; } public string Name { get; set; } public virtual ICollection<Student> Students { get; set; } } }And following is the code for the Student model.
using System.ComponentModel.DataAnnotations.Schema; namespace CascadingDropdownEF.Models { public class Student { public int StudentId { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public int StandardId { get; set; } [ForeignKey("StandardId")] public Standard Standard { get; set; } } }Now once we have our model ready it’s time to create our Entity framework context class. We are going to use Entity code first model here . So here is the code for the Entity framework context.
using System.Data.Entity; using System.Data.Entity.ModelConfiguration.Conventions; namespace CascadingDropdownEF.Models { public class StudentContext : DbContext { public StudentContext() : base("name=StudentConnectionString") { } public DbSet<Standard> Standards { get; set; } public DbSet<Student> Students { get; set; } protected override void OnModelCreating(DbModelBuilder modelBuilder) { modelBuilder.Conventions.Remove<PluralizingTableNameConvention>(); } } }Here I have created two DbSet for students and standards. Also in model creating event I have removed plural names so when entity framework create tables when we run this application it will remove plural names from the table.
Creating Web Forms and using Entity framework for cascading dropdowns:

After creating Web Form I have written following HTML code in aspx file.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="CascadingDrodownDemo.aspx.cs" Inherits="CascadingDropdownEF.CascadingDrodownDemo" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>Cascading dropwon demo</title> </head> <body> <form id="form1" runat="server"> <div> <asp:DropDownList runat="server" ID="ddlStandard" CssClass="dropdown" AutoPostBack="true" OnSelectedIndexChanged="ddlStandard_OnSelectedIndexChanged"/> </div> <br/> <div> <asp:DropDownList runat="server" ID="ddlStudent" CssClass="dropdown"/> </div> </form> </body> </html>You can see that I have created two ASP.NET dropdowns Standard and Student and Also for Standard dropdown I have autopostback=”true” and selected index change event.
On the aspx.cs file i have written the following code.
using System; using System.Linq; using CascadingDropdownEF.Models; namespace CascadingDropdownEF { public partial class CascadingDrodownDemo : System.Web.UI.Page { private readonly StudentContext _studentContext; public CascadingDrodownDemo() { _studentContext = new StudentContext(); } protected void Page_Load(object sender, EventArgs e) { if (!Page.IsPostBack) { BindStandards(); BindStudents(); } } private void BindStudents(int? standardId=null) { ddlStudent.Items.Clear(); if (standardId != null) { var students = from student in _studentContext.Students where student.StandardId == standardId.Value select new { StudentId = student.StudentId, Name = student.FirstName + " " + student.LastName }; ddlStudent.DataSource = students.ToList(); ddlStudent.DataTextField = "Name"; ddlStudent.DataValueField = "StudentId"; ddlStudent.DataBind(); } ddlStudent.Items.Insert(0,"--Select Student--"); } private void BindStandards() { ddlStudent.Items.Clear(); var standards = _studentContext.Standards.ToList(); ddlStandard.DataSource = standards; ddlStandard.DataTextField = "Name"; ddlStandard.DataValueField = "StandardId"; ddlStandard.DataBind(); ddlStandard.Items.Insert(0,"--Select Standard--"); } protected void ddlStandard_OnSelectedIndexChanged(object sender, EventArgs e) { BindStudents(Convert.ToInt32(ddlStandard.SelectedValue)); } } }Here You can see that I have created two method BindStudent and BindStarnds. In Bindstandards it fetches all the standards and bind it to Standard dropdown . In BindStudents method, I have used a nullable parameter standardId which will be null by default. So If standard id is not provided as you can see it will only insert default item. Now in page load method, I have called this two methods. Also, you can see that I have written Standard event dropdown selected index change event which will pass standard value.
Now everything is ready. I have inserted following data in Standard and Student table.

And student table like following.

Now when you run the application. It will look like following.
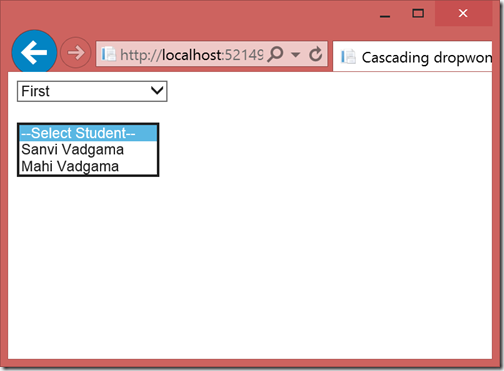
That’s it. Hope you like it. Stay tuned for more.
You can find complete source code of this application at following location on github- https://github.com/dotnetjalps/CascadingDropdownEF