As I have already posted about the how to fetch data in my earlier post for Dapper ORM. In this post I am going to explain how we can insert data with the dapper ORM. So let’s extend the earlier post project. As explained in earlier post I have already created a class called CustomerDB this class will contains all the operation with Dapper Micro ORM. So For inserting data let’s first create CREATE method like following in CutomerDB Class like following. In that I have create a simple Insert Query in string and then using connection.execute method to execute method. Following is code for that.
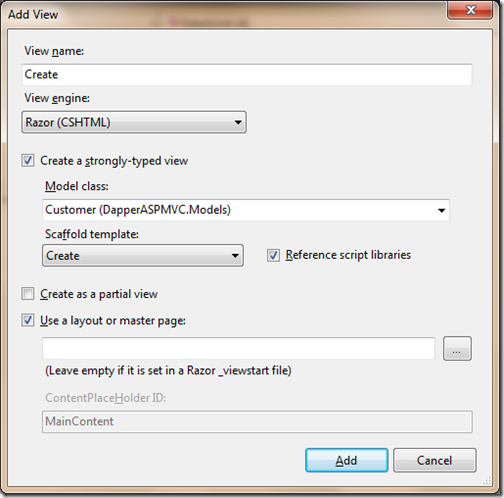
That’s it now we are ready. Now let’s test it in browser. Like following.
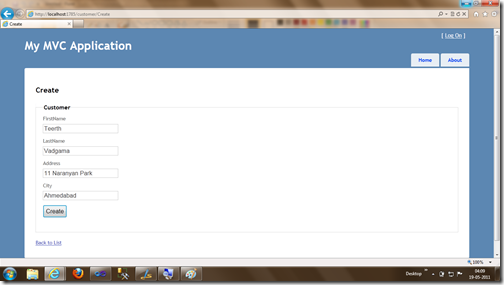
Now let’s click and create and then it will redirect us to customer list page like following. Where you can see the newly added details.
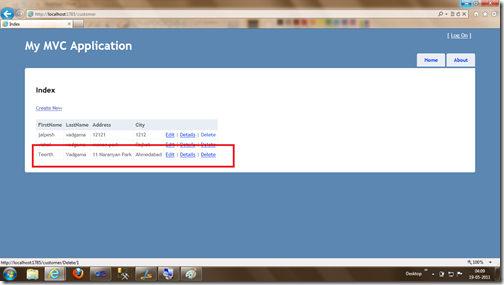
So that’s it. Its very easy to Insert data with Dapper Micro ORM. Hope you like it…Stat tuned for more.
Note: For reference of this post you can also see my first post called -Playing with dapper Micro ORM and ASP.NET MVC 3.0.

public class CustomerDB { public string Connectionstring = @"Data Source=DotNetJalps\SQLExpress;Initial Catalog=CodeBase;Integrated Security=True"; public IEnumerable<Customer> GetCustomers() { using (System.Data.SqlClient.SqlConnection sqlConnection = new System.Data.SqlClient.SqlConnection(Connectionstring)) { sqlConnection.Open(); var customer = sqlConnection.Query<Customer>("Select * from Customer"); return customer; } } public string Create(Customer customerEntity) { try { using (System.Data.SqlClient.SqlConnection sqlConnection = new System.Data.SqlClient.SqlConnection(Connectionstring)) { sqlConnection.Open(); string sqlQuery = "INSERT INTO [dbo].[Customer]([FirstName],[LastName],[Address],[City]) VALUES (@FirstName,@LastName,@Address,@City)"; sqlConnection.Execute(sqlQuery, new { customerEntity.FirstName, customerEntity.LastName, customerEntity.Address, customerEntity.City }); sqlConnection.Execute(sqlQuery); sqlConnection.Close(); } return "Created"; } catch (Exception ex) { return ex.Message; } } }Now we are ready with Create Method for database now let’s create two ActionResult for the Creating customer like following in Customer Controller like following.
public ActionResult Create() { return View(); } // // POST: /Customer/Create [HttpPost] public ActionResult Create(Customer customer) { try { // TODO: Add insert logic here var customerEntities = new CustomerDB(); customerEntities.Create(customer); return RedirectToAction("Index"); } catch { return View(); } }Now we are ready with the both ActionResult. First ActionResult will return simple view of Create which we are going to create now and another ActionResult Create will get customer object from the form submitted and will call our create method of CustomerDB Class. Now it’s time to create a view for adding customer. Right Click return view Statement in Create Action Result and Click Add View and Just Create view like following.
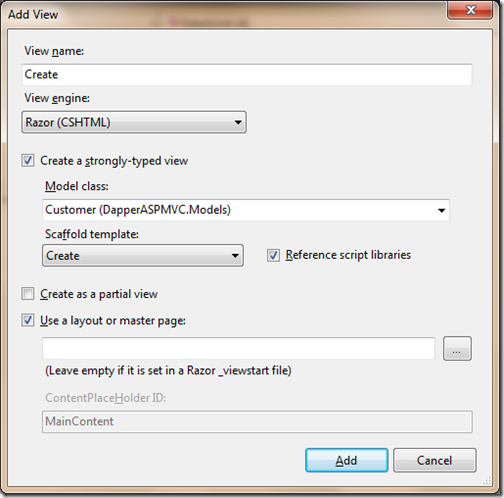
That’s it now we are ready. Now let’s test it in browser. Like following.
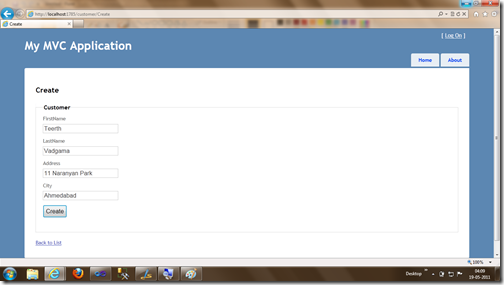
Now let’s click and create and then it will redirect us to customer list page like following. Where you can see the newly added details.
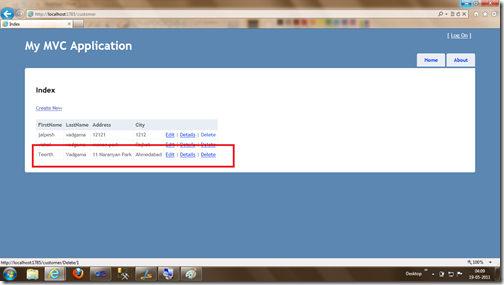
So that’s it. Its very easy to Insert data with Dapper Micro ORM. Hope you like it…Stat tuned for more.
Note: For reference of this post you can also see my first post called -Playing with dapper Micro ORM and ASP.NET MVC 3.0.