Calling a web service from JavaScript was easy task earlier you need to create a proxy class for JavaScript and then you need to write lots of java script but with Microsoft ASP.NET Ajax you can call web services from JavaScript very easily with some line of JavaScript and even better you can also handle the errors if web service failed to return result. Let’s create a simple hello world web service which will print Hello world string and then we will call that web service into JavaScript. Following is a code for web service.
namespace DotNetJapsSocial
{
/// <summary>
/// Summary description for HelloWorld
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class HelloWorld : System.Web.Services.WebService
{
[WebMethod]
public string PrintHelloWolrdMessage()
{
return "Hello World";
}
}
}
Here we have created a web method called
PrintHelloWorldMessage which will return a string “Hello world”. Please see the [System.Web.Script.Services.ScriptService] attribute of HelloWorld Service class which will enable web service to invoked by any ECMA Script and here we are using JavaScript to call web service.
Now lets create three functions in JavaScript
CallWebService,
ReuqestCompleteCallback and
RequestFailedCallback. Following is the code for that.
<script type="text/javascript">
function CallWebService() {
DotNetJapsSocial.HelloWorld.PrintHelloWolrdMessage(ReuqestCompleteCallback, RequestFailedCallback);
}
function ReuqestCompleteCallback(result)
{
// Display the result.
var divResult = document.getElementById("divMessage");
divResult.innerHTML = result;
}
function RequestFailedCallback(error) {
var stackTrace = error.get_stackTrace();
var message = error.get_message();
var statusCode = error.get_statusCode();
var exceptionType = error.get_exceptionType();
var timedout = error.get_timedOut();
// Display the error.
var divResult = document.getElementById("Results");
divResult.innerHTML = "Stack Trace: " + stackTrace + "<br/>" +
"Service Error: " + message + "<br/>" +
"Status Code: " + statusCode + "<br/>" +
"Exception Type: " + exceptionType + "<br/>" +
"Timedout: " + timedout;
}
</script>
The CallWebService function will call the web service method. Here we have to pass web service method will complete path like DotNetJapsSocial.HelloWorld.PrintHelloWolrdMessage. The another function ReuqestCompleteCallback will return result call back of web service as parameter in our case it will be a string. Another function RequestFailedCallback is failed callback of web service. If web service returns some error then it will goes to that function. So here you can handle errors.
Now Let’s Create div which will print message and a button to call web service like following.
<div>
<input type="button" value="Call Web Service"
onclick="CallWebService();"/>
</div>
<div id="divMessage">
</div>
so when you click button then it will crate output like following.
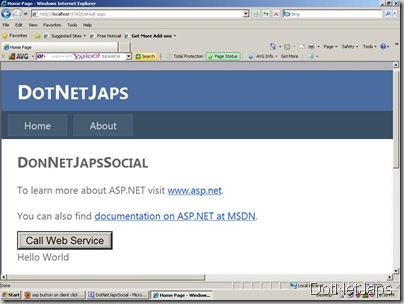
Hope this will help you..Happy coding..
