As you know with jQuery selectors we can have single selection but lots of people does not know that jQuery is also provide multiple selection also. Let’s take an example. You have following html structure.
How to to select all p in div with jQuery
As you know with jQuery selectors we can have single selection but lots of people does not know that jQuery is also provide multiple selection also. Let’s take an example. You have following html structure.
Extending jQuery with jQuery.Extend
http://api.jquery.com/jQuery.extend/
It merges the contents of two or more objects together into the first object.
How to call ASP.Net page method with jQuery
What is ASP.Net Page Method?
Creating a page method:
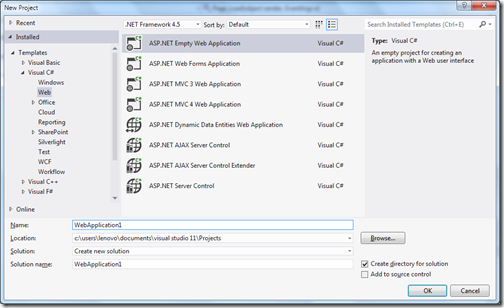
How to consume ASP.NET WebAPI from jQuery
In this post I am going to explain how to consume ASP.NET WebAPI from jQuery.
Creating a basic WebAPI:
To create a web API you need to install ASP.NET MVC 4.0 and then you create a new project for MVC .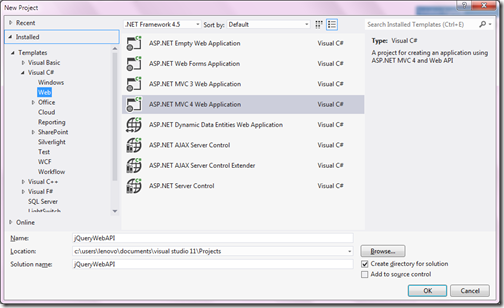
Now once you click Ok. It will ask for type of project. You want to create you need to select Web API there.
How to hide title bar in jQuery UI modal dialog?
Here in the above code you can see I have create a hello world pop up with asp.net jQuery CDN.
<html> <head> <title>Hello World Popup</title> <link type="text/css" href="http://ajax.aspnetcdn.com/ajax/jquery.ui/1.10.0/themes/smoothness/jquery-ui.css" rel="stylesheet" /> <script language="javascript" src="http://ajax.aspnetcdn.com/ajax/jQuery/jquery-1.9.0.min.js"></script> <script language="javascript" src="http://ajax.aspnetcdn.com/ajax/jquery.ui/1.10.0/jquery-ui.min.js"></script> <script type="text/javascript"> function Show() { $("#dialog:ui-dialog").dialog("destroy"); $("#dialog-modal").dialog({ height: 300, width: 200, modal: true }); } </script> </head> <body> <div id="dialog-modal" title="Hello Word" style="display: none"> Hello World Juqry UI popup </div> <input type="button" onclick="javascript: Show()" value="click me" /> </body> </html>
Async file upload with jquery and ASP.NET
So I have download that plug from the following link. This plug in created by yvind Saltvik
After downloading the plugin and going through it documentation I have found that I need to write a page or generic handler which can directly upload the file on the server. So I have decided to write a generic handler for that as generic handler is best suited with this kind of situation and we don’t have to bother about response generated by it.
So let’s create example and In this example I will show how we can create async file upload without writing so much code with the help of this plugin. So I have create project called JuqeryFileUpload and our need for this example to create a generic handler. So let’s create a generic handler. You can create a new generic handler via right project-> Add –>New item->Generic handler just like following.
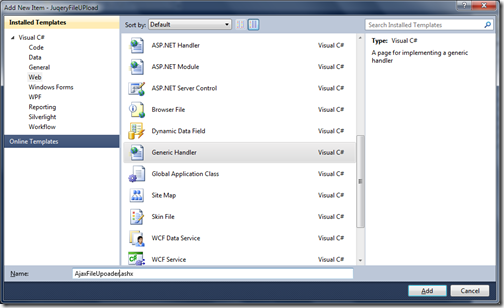
I have created generic handler called AjaxFileuploader and following is simple code for that.
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.IO; namespace JuqeryFileUPload { /// <summary> /// Summary description for AjaxFileUploader /// </summary> public class AjaxFileUploader : IHttpHandler { public void ProcessRequest(HttpContext context) { if (context.Request.Files.Count > 0) { string path = context.Server.MapPath("~/Temp"); if (!Directory.Exists(path)) Directory.CreateDirectory(path); var file = context.Request.Files[0]; string fileName; if (HttpContext.Current.Request.Browser.Browser.ToUpper() == "IE") { string[] files = file.FileName.Split(new char[] { '\\' }); fileName = files[files.Length - 1]; } else { fileName = file.FileName; } string strFileName=fileName ; fileName = Path.Combine(path, fileName); file.SaveAs(fileName); string msg = "{"; msg += string.Format("error:'{0}',\n", string.Empty); msg += string.Format("msg:'{0}'\n", strFileName); msg += "}"; context.Response.Write(msg); } } public bool IsReusable { get { return true; } } } }
As you can see in above code.I have written a simple code to upload a file from received from file upload plugin into the temp directory on the server and if this directory is not there on the server then it will also get created by the this generic handler.At the end of the of execution I am returning the simple response which is required by plugin itself. Here in message part I am passing the name of file uploaded and in error message you can pass error if anything occurred for the time being I have not used right now.
As like all jQuery plugin this plugin also does need jQuery file and there is another .js file given for plugin called ajaxfileupload.js. So I have created a test.aspx to test jQuery file and written following html for that .
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Test.aspx.cs" Inherits="JuqeryFileUPload.Test" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <script type="text/javascript" src="jquery.js"></script> <script type="text/javascript" src="ajaxfileupload.js"></script> </head> <body> <form id="form1" runat="server"> <div> <input id="fileToUpload" type="file" size="45" name="fileToUpload" class="input"> <button id="buttonUpload" onclick="return ajaxFileUpload();">Upload</button> <img id="loading" src="loading.gif" style="display:none;"> </div> </form> </body> </html>
As you can see in above code there its very simple. I have included the jQuery and ajafileupload.js given by the file upload give and there are three elements that I have used one if plain file input control you can also use the asp.net file upload control and but here I don’t need it so I have user file upload control. There is button there called which is calling a JavaScript function called “ajaxFileUpload” and here we will write a code upload that. There is an image called loading which just an animated gif which will display during the async call of generic handler. Following is code ajaxFileUpload function.
<script type="text/javascript"> function ajaxFileUpload() { $("#loading") .ajaxStart(function () { $(this).show(); }) .ajaxComplete(function () { $(this).hide(); }); $.ajaxFileUpload ( { url: 'AjaxFileUploader.ashx', secureuri: false, fileElementId: 'fileToUpload', dataType: 'json', data: { name: 'logan', id: 'id' }, success: function (data, status) { if (typeof (data.error) != 'undefined') { if (data.error != '') { alert(data.error); } else { alert(data.msg); } } }, error: function (data, status, e) { alert(e); } } ) return false; } </script>
As you can see in above code I have putted our generic handler url which will upload the file on server as url parameter. There is also parameter called secureURI is required to be true if you are uploading file through the secure channel and as a third parameter we have to pass a file upload control id which I have already passed and in fourth parameter we have to passed busy indicator which I have also passed. Once we passed all the parameter then it will call a method for plugin and will return response in terms of message and error. So There is two handler function written for that.
That’s it. Our async file upload is ready. As you can easily integrate it and also it working fine in all the browser. Hope you like it. Stay tuned for more. Till then happy programming..
Jquery and ASP.NET- Set and Get Value of Server control
For this I am going to take one simple example asp.net page which contain two textboxes txtName and txtCopyName and button called copyname. On click of that button it will get the value from txtName textbox and set value of another box. So following is HTML for this.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Index.aspx.cs" Inherits="Experiment.Index" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:TextBox ID="txtName" runat="server" /> <Br /> <asp:TextBox ID="txtCopyName" runat="server"/> <Br /> <asp:button ID="btnCopyName" runat="server" Text="Copy" OnClientClick="javascript:CopyName();return false;"/> </div> </form> </body> </html>
As you can see in following code there are two textbox and one button which will call JavaScript function called to CopyName to copy text from one textbox from another textbox.Now we are going to use the Jquery for this. So first we need to include Jquery script file to accomplish the task. So I am going link that jquery.js file in my header section like following.
<head > <title></title> <script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.6.2.js"></script> </head>
Here I have used the ASP.NET Jquery CDN. If you want know more about Jquery CDN you can visit this link.
http://www.asp.net/ajaxlibrary/cdn.ashx
Now it’s time to write query code. Here I have used val function to set and get value for the element. Following is the code for CopyName function.
function CopyName() { var name = $("#<%=txtName.ClientID%>").val(); //get value $("#<%=txtCopyName.ClientID%>").val(name); //set value return false; }
Here I have used val function of jquery to set and get value. As you can see in the above code, In first statement I have get value in name variable and in another statement it was set to txtCopy textbox. Some people might argue why you have used that ClientID but it’s a good practice to have that because when you use multiple user controls your id and client id will be different. From this I have came to know that there are lots of people still there who does not basic Jquery things so in future I am going to post more post on jquery basics.That’s it. Hope you like it.Stay tuned for more.. Happy programming and Namaste!!
JQuery UI 1.8.9 new version launch today
JQuery UI contains great controls and it’s very useful when developing sites. Today a new version of Jquery UI is launched.
You can find more details about that from following link.
http://blog.jqueryui.com/2011/01/jquery-ui-1-8-9/
It’s contains Bug fixes for Datepicker, Tabs and other things and also contains some localization improvements on the date picker.
Calling an asp.net web service from jQuery
Let’s create a simple Hello World web service. Go to your project right click->Add –> New Item and select web service and add a web service like following.
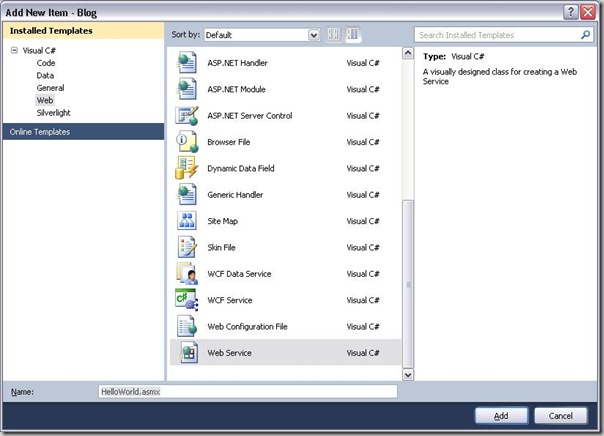
Now modify the code of web service like following.
[WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] [System.ComponentModel.ToolboxItem(false)] // To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line. [System.Web.Script.Services.ScriptService] public class HelloWorld : System.Web.Services.WebService { [WebMethod] public string PrintMessage() { return "Hello World..This is from webservice"; } }
Here please make sure that [System.Web.Script.Services.ScriptService] is un commented because this attribute is responsible for allowing web service to be called by Client side scripts.
Now to call this web service from jquery we have to include jQuery Js like following. I am already having in my project so i don’t need to include in project just need to add script tag like following.
<script type="text/javascript" src="Scripts/jquery-1.4.1.min.js"> </script>
Now let’s add some JavaScript code to call web service methods like following.
<script language="javascript" type="text/javascript"> function CallWebServiceFromJquery() { $.ajax({ type: "POST", url: "HelloWorld.asmx/PrintMessage", data: "{}", contentType: "application/json; charset=utf-8", dataType: "json", success: OnSuccess, error: OnError }); } function OnSuccess(data, status) { alert(data.d); } function OnError(request, status, error) { alert(request.statusText); } </script>
Here I have written three functions in JavaScript First one is CallWebserviceFromJquery which will call the web service. The another two functions are delegates of webservice if Success is there then onSuccess Method will be called and If Error is there then OnError method will be called.
Inside the CallWebserviceFromJquery I have passed some parameter which will call web service with Ajax capabilities of $ jQuery object. Here are the description for each parameter.
Type: This can be GET or POST for web service we have to take POST as by default web service does not work with GET to prevent Cross site requests.
Url: Here will be url of the webservice. You have insert fully qualified web service name here.
Data:Here it will be Empty as we not calling web service with parameters if you are calling web service with parameter then you have to pass that here.
ContentType: here you have to specify what type of content is going to return by web service.
Datatype:Json it will be result type.
Sucess:Here I have called the OnSuccess when the call is complete successfully. If you check the OnSuccess method you will see that I have set the returned result from the web service to the label. In the OnSuccess method body you see ‘data.d’. The ‘d’ here is the short form of data.
Error: Same as I have done with OnSuccess. If any error occurred while retrieving the data then the OnError method is invoked.
Now let’s add a asp.net button for on client click we will call the javascript function like following.
<asp:Button ID="btnCallWebService" runat="server" OnClientClick="CallWebServiceFromJquery()" Text="Call Webservice"/>
Now Let’s run it and here is the output in browser.
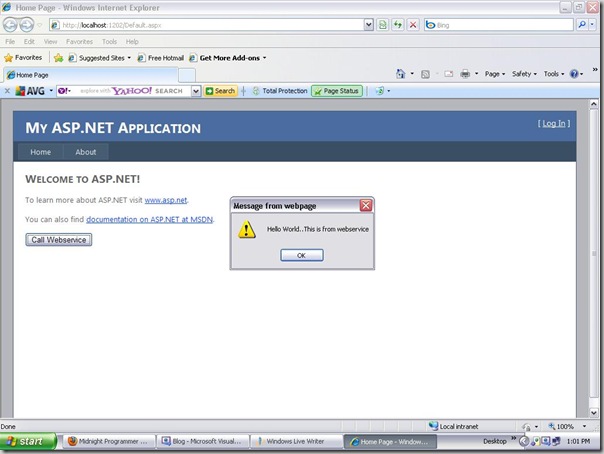
Hope this will help you!!!.. Happy programming..
jQuery- JavaScript Library Write less do more
This is introductory post jQuery an open source JavaScript library. I know what you guys thinking and I also know that jQuery does not required introduction. It is so much popular and most of web developers whether they are developing using asp.net,php,Jsp or any language on web they are using jQuery but this post is for who is not aware of it and or they are new to the web based programming.
We all are using JavaScript for client side scripting language almost 95% percentage of web application uses JavaScript as client script. We do lots of things with JavaScript right from the alerts to validation of controls to animation and now days we calling web services from JavaScript and fetching server side data also and rendering them into browser. If you are using JavaScript then you are following some patterns like selecting a element on and then perform some operation on that element on client side like do validation of controls or changing the style sheet of that element or animating them etc. jQuery help you doing this in rather simpler way so you can save lots of time doing other things instead of writing long complex JavaScript.
Why jQuery?
jQuery is a fast and concise JavaScript Library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. jQuery is designed to change the way that you write JavaScript. jQuery provides lots of functionality like animating elements,Ajax interaction with server sides web service, changing CSS of a Elements etc and you can complete those task in minimum line of the code. For example let’s see the code which are given Homepage of jQuery website.
$("p.neat").addClass("ohmy").show("slow");
Use Full Links For jQuery
If you want to learn jQuery then following are links which can be useful to you.http://docs.jquery.com/How_jQuery_Works- For How jQuery Works
http://jquery.org/history- For History of jQuery
http://docs.jquery.com/Main_Page- jQuery documentation
Hope this will help you!!! Happy programming..