Before some day one of my friend asked me to install NuGet in project another then startup project. At that time I don’t have any idea about it. So I did some R and D on web and found one easiest way to do it. So let’s first create a solution where we have more then one project in single solution. I have created a new solution and create three project in single solution as below.
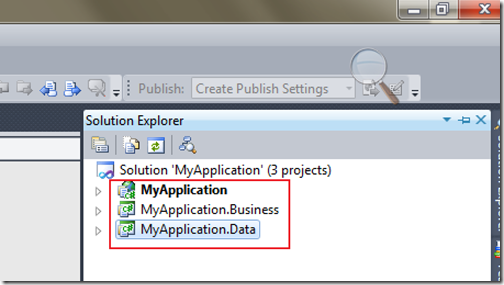
Now I want to add EFCodeFirst NuGet package to MyApplication.Data project as I want to use entity framework code first for database operations. To add new package with in the project. you can select project and then click ‘Manage NuGet Packages’ like following.
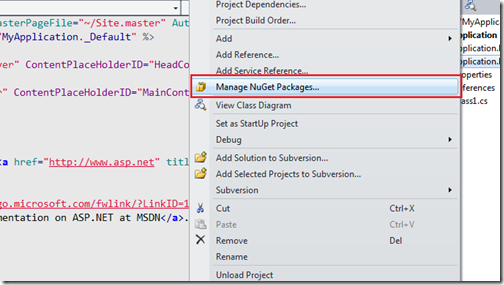
Once you click it will show a dialog for NuGet Packages like following.
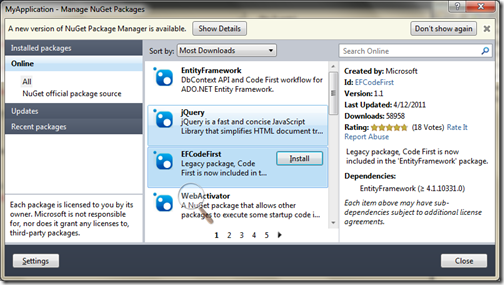
I have selected EFCodeFirst and Once I clicked ‘Install’ . It has added reference to MyApplication.Data Project like following.
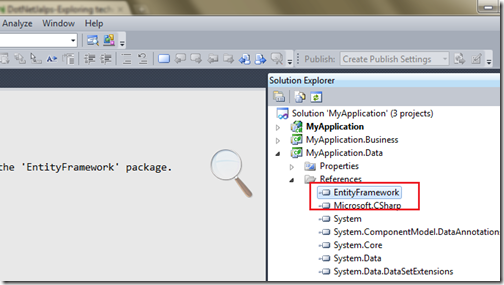
That’s it. It’s very easy. Hope you like it..Stay tuned for more.. Till then happy programming and Namaste!!!.
- MyApplication- Main web application project.
- MyApplication.Business- This project contains all business logic classes of application
- MyApplication.Data- This project contains all data access layers classes of application
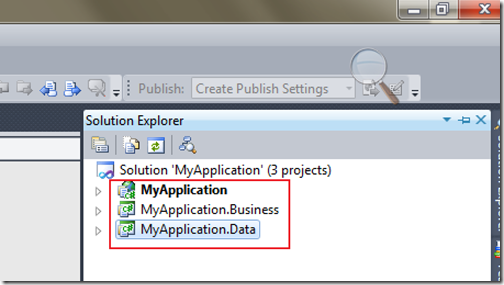
Now I want to add EFCodeFirst NuGet package to MyApplication.Data project as I want to use entity framework code first for database operations. To add new package with in the project. you can select project and then click ‘Manage NuGet Packages’ like following.
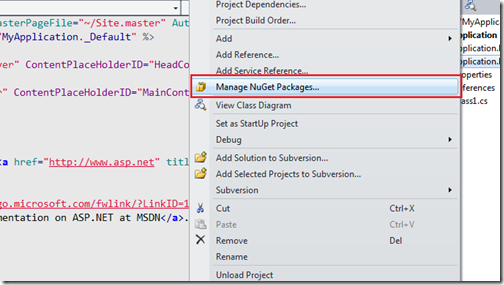
Once you click it will show a dialog for NuGet Packages like following.
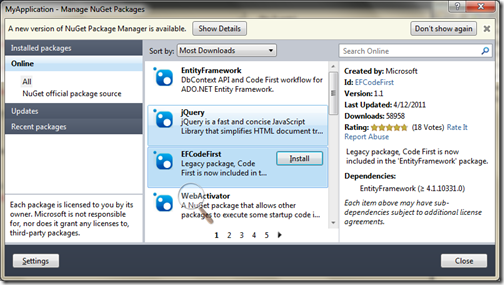
I have selected EFCodeFirst and Once I clicked ‘Install’ . It has added reference to MyApplication.Data Project like following.
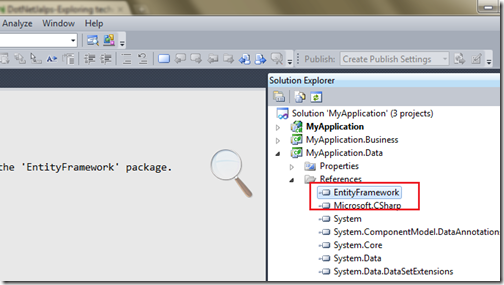
That’s it. It’s very easy. Hope you like it..Stay tuned for more.. Till then happy programming and Namaste!!!.