Variable Reference Code Highlighting
It’s a new feature there in Visual studio 2010. Whenever you put the cursor on the variable it will highlight all the code where that variable used. This is a very nice features as within one shot we can see what is done with that variable in code just like following.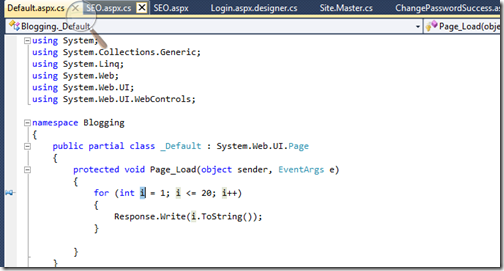
Hiding selected code in Visual Studio 2010
In earlier version of Microsoft Visual Studio We can collapse the method and region. But you can not collapse the particular code block but with Visual Studio 2010 code you can hide the particular selected code via right click outlining –>Hide Selection and you can highlight code lets see first following code.using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace Blogging { public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (Page.IsPostBack) { Response.Write("Page is not postback"); } for (int i = 1; i <= 20; i++) { Response.Write(i.ToString()); } } } }
Now I want to hide the following Page.IsPostBack part via right click Outlining –> Hide Selection
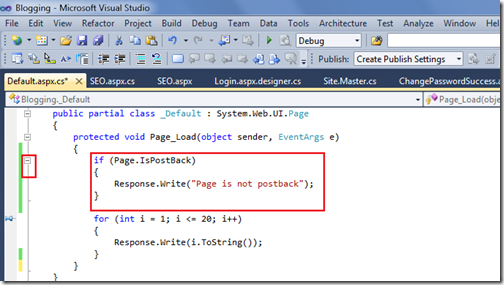
As you can see in above image there is –(Minus) Icon so you can collapse that code Just like region. Now you want to undo the collapsing then once again you need to select the code in editor and then right click and then select Outlining->Stop Hiding Current and then it will be treated as normal code with out collapsible block Like below.
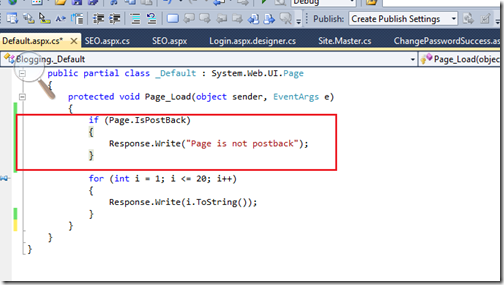
That’s it hope you liked it.. Stay tuned for more.. Happy programming..