This blog post is part of C# 6.0 Features Series.Microsoft announced the new version of C# 6.0 at the day of visual studio connect event on November. They have not added any big features to C# 6.0 but they have listened to community and added few small features which comes really handy. One of the that is nameof operator. In this blog post we learn why nameof operator is very useful.
Let’s create a class Employee with few properties.
public class Employee { public string FirstName { get; set; } public string LastName { get; set; } }Now what I want to do here is to create a object with object initializer and print values of properties with print method.
Old way of doing this to pass hardcoded string like following.
class Program { static void Main(string[] args) { Employee employee = new Employee { FirstName = "Jalpesh", LastName = "Vadgama" }; Print(null); } static void Print(Employee employee) { if (employee == null) throw new ArgumentException("employee"); Console.WriteLine(employee.FirstName); Console.WriteLine(employee.LastName); } }Here strings are hardcoded in argument exception so that we have problem because when change names of class we have to change this hardcoded string also.
With nameof operator:
class Program { static void Main(string[] args) { Employee employee = new Employee { FirstName = "Jalpesh", LastName = "Vadgama" }; Print(null); } static void Print(Employee employee) { if (employee == null) throw new ArgumentException(nameof(employee)); Console.WriteLine(employee.FirstName); Console.WriteLine(employee.LastName); } }Here you can see that I have used nameof operator in place of hardcoded string so now even if we change the name of class then also we don’t have to worry about it. Now let’s run this and we will see output as expected as we have passed null to print method.
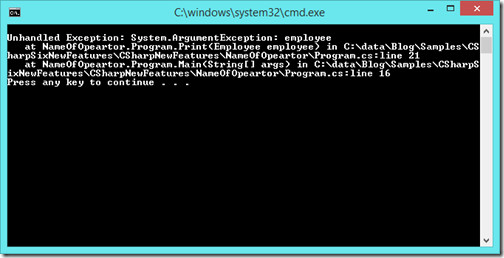
Here we have passed null so it will throw argument exception. You can find complete source code on github at following location.
https://github.com/dotnetjalps/Csharp6NewFeatures
That’s it. Hope you like it. Stay tuned for more!.
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.