As I have post it in earlier post that jQuery is one of most popular JavaScript library in the world amongst web developers Lets take a example calling ASP.NET web service with jQuery . You will see at the end of the example that how easy it is to call a web service from the jQuery.
Let’s create a simple Hello World web service. Go to your project right click->Add –> New Item and select web service and add a web service like following.
Now modify the code of web service like following.
Here please make sure that [System.Web.Script.Services.ScriptService] is un commented because this attribute is responsible for allowing web service to be called by Client side scripts.
Now to call this web service from jquery we have to include jQuery Js like following. I am already having in my project so i don’t need to include in project just need to add script tag like following.
Now let’s add some JavaScript code to call web service methods like following.
Here I have written three functions in JavaScript First one is CallWebserviceFromJquery which will call the web service. The another two functions are delegates of webservice if Success is there then onSuccess Method will be called and If Error is there then OnError method will be called.
Inside the CallWebserviceFromJquery I have passed some parameter which will call web service with Ajax capabilities of $ jQuery object. Here are the description for each parameter.
Type: This can be GET or POST for web service we have to take POST as by default web service does not work with GET to prevent Cross site requests.
Url: Here will be url of the webservice. You have insert fully qualified web service name here.
Data:Here it will be Empty as we not calling web service with parameters if you are calling web service with parameter then you have to pass that here.
ContentType: here you have to specify what type of content is going to return by web service.
Datatype:Json it will be result type.
Sucess:Here I have called the OnSuccess when the call is complete successfully. If you check the OnSuccess method you will see that I have set the returned result from the web service to the label. In the OnSuccess method body you see ‘data.d’. The ‘d’ here is the short form of data.
Error: Same as I have done with OnSuccess. If any error occurred while retrieving the data then the OnError method is invoked.
Now let’s add a asp.net button for on client click we will call the javascript function like following.
Now Let’s run it and here is the output in browser.
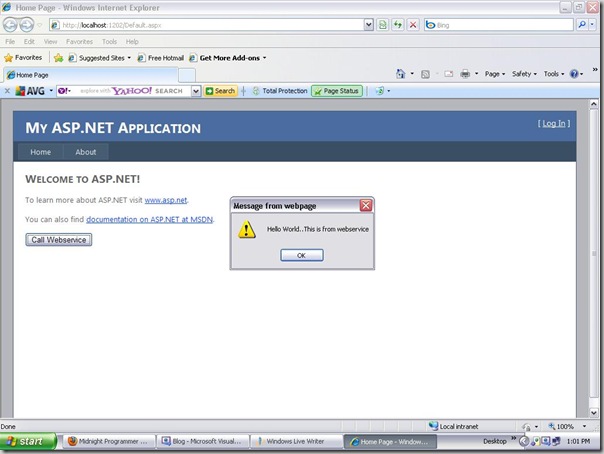
Hope this will help you!!!.. Happy programming..
Let’s create a simple Hello World web service. Go to your project right click->Add –> New Item and select web service and add a web service like following.
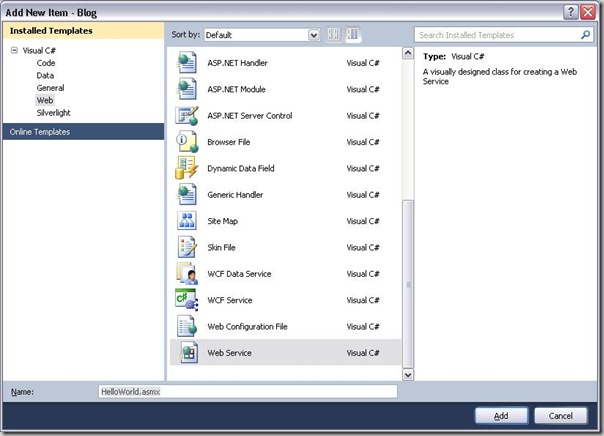
Now modify the code of web service like following.
[WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] [System.ComponentModel.ToolboxItem(false)] // To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line. [System.Web.Script.Services.ScriptService] public class HelloWorld : System.Web.Services.WebService { [WebMethod] public string PrintMessage() { return "Hello World..This is from webservice"; } }
Here please make sure that [System.Web.Script.Services.ScriptService] is un commented because this attribute is responsible for allowing web service to be called by Client side scripts.
Now to call this web service from jquery we have to include jQuery Js like following. I am already having in my project so i don’t need to include in project just need to add script tag like following.
<script type="text/javascript" src="Scripts/jquery-1.4.1.min.js"> </script>
Now let’s add some JavaScript code to call web service methods like following.
<script language="javascript" type="text/javascript"> function CallWebServiceFromJquery() { $.ajax({ type: "POST", url: "HelloWorld.asmx/PrintMessage", data: "{}", contentType: "application/json; charset=utf-8", dataType: "json", success: OnSuccess, error: OnError }); } function OnSuccess(data, status) { alert(data.d); } function OnError(request, status, error) { alert(request.statusText); } </script>
Here I have written three functions in JavaScript First one is CallWebserviceFromJquery which will call the web service. The another two functions are delegates of webservice if Success is there then onSuccess Method will be called and If Error is there then OnError method will be called.
Inside the CallWebserviceFromJquery I have passed some parameter which will call web service with Ajax capabilities of $ jQuery object. Here are the description for each parameter.
Type: This can be GET or POST for web service we have to take POST as by default web service does not work with GET to prevent Cross site requests.
Url: Here will be url of the webservice. You have insert fully qualified web service name here.
Data:Here it will be Empty as we not calling web service with parameters if you are calling web service with parameter then you have to pass that here.
ContentType: here you have to specify what type of content is going to return by web service.
Datatype:Json it will be result type.
Sucess:Here I have called the OnSuccess when the call is complete successfully. If you check the OnSuccess method you will see that I have set the returned result from the web service to the label. In the OnSuccess method body you see ‘data.d’. The ‘d’ here is the short form of data.
Error: Same as I have done with OnSuccess. If any error occurred while retrieving the data then the OnError method is invoked.
Now let’s add a asp.net button for on client click we will call the javascript function like following.
<asp:Button ID="btnCallWebService" runat="server" OnClientClick="CallWebServiceFromJquery()" Text="Call Webservice"/>
Now Let’s run it and here is the output in browser.
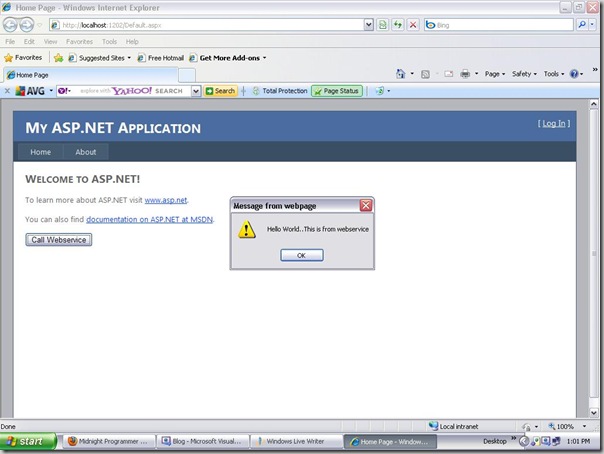
Hope this will help you!!!.. Happy programming..
I recently came across your blog and have been reading along. I thought I would leave my first comment. I don't know what to say except that I have enjoyed reading. Nice blog. I will keep visiting this blog very often.
ReplyDeleteasp dot net programming
Hi jalpesh
ReplyDeleteI came across this post of your blog while i was searching for issue with calling a webservice from javascript.I appreciate this post as it proved a ready reckoner for me.
Everyting worked fine only in IE.The onsuccess function is not been invoked despite the fact that the webservice had sent a succesful response.I could see the response in the firebug.
Please help me out of this.
Thanks in advance
@Ramoji.
ReplyDeleteCan you send me your code and which version you are using of Jquery.
Regards,
Jalpesh
This is very nice blog because information provided here
ReplyDeletethrough the article and the pictures are very effective. Because sometimes
words cannot explain the things that pictures can and here the words and
pictures both are expressing the things in balance.
Thanks Windows Hosting!!
ReplyDeleteThis comment has been removed by a blog administrator.
ReplyDeleteI recently came across this blog informative post to all Asp.net , j Query developers those are in learning process .
ReplyDeleteGreat post thank you
You're welcome
Delete