In this blog post we are going to learn about how we can call asp.net page methods from jQuery.
Page methods are available from ASP.Net 2.0. It’s an alternative to web services in ASP.Net application. This methods are exposed at client side and you can call that page methods directly from JavaScript.It is an easy way to communicate asynchronously with the server using Ajax.
So for creating a page method let’s create a asp.net web empty web application.
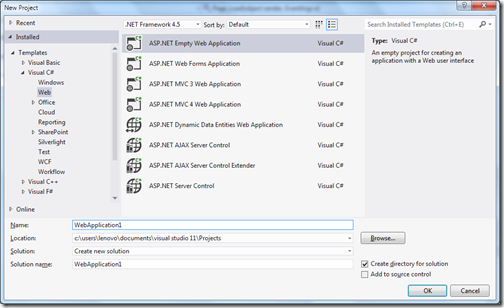
Now we are ready with our application let’s write a simple hello world page method.
Calling page method from jQuery:
Now our HTML and server side code is ready. So it’s time to write some JavaScript code. Following is a code for calling a page method from JavaScript.
Here in the above code you have seen that I have written code in document ready function of jQuery which will called when page will be loaded and with jQuery Ajax function I have called page method and replace the text of div with the reply from Page method. Now when you run this in browser. It’s look like following.
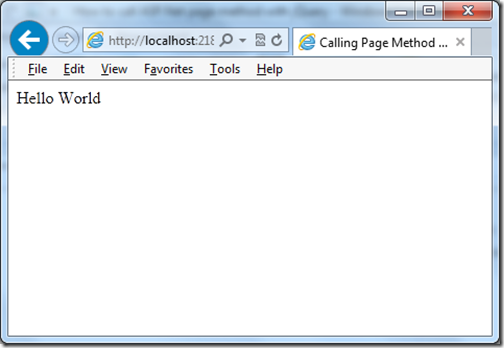
You can see It’s a very easy to call a page method in jQuery. Hope you like it. Stay tuned for more..
What is ASP.Net Page Method?
Creating a page method:
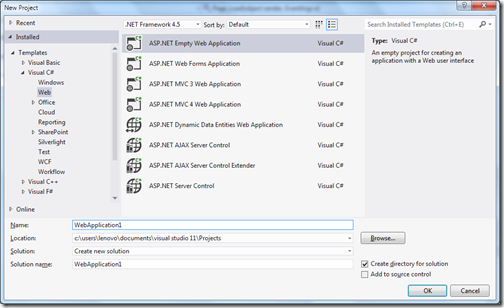
Now we are ready with our application let’s write a simple hello world page method.
using System; using System.Web.Services; namespace WebApplication1 { public partial class WebForm1 : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } [WebMethod] public static string HelloWorld() { return "Hello World"; } } }Now our server side code is ready It’s time to write some code for html.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.WebForm1" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>Calling Page Method with jquery</title> <script type="text/javascript" src="Scripts/jquery-2.0.0.min.js"></script> </head> <body> <form id="form1" runat="server"> <asp:ScriptManager ID="scriptManager" runat="server" EnablePageMethods="true"> </asp:ScriptManager> <div id="HelloWorldContainer"> </div> </form> </body> </html>Now in the above HTML code you have seen we have included the jQuery min JavaScript file with script tag and then I have created a script manager with EnabledPageMethods as true. Please note that scriptmanager is required here to call page methods from JavaScript. Also I have created a div with Id “HelloWorldContainer” to print output of HelloWorld page method.
Calling page method from jQuery:
Now our HTML and server side code is ready. So it’s time to write some JavaScript code. Following is a code for calling a page method from JavaScript.
<script type="text/javascript"> $(document).ready(function () { $.ajax({ type: "POST", url: "WebForm1.aspx/HelloWorld", data: "{}", contentType: "application/json; charset=utf-8", dataType: "json", success: function (msg) { $("#HelloWorldContainer").text(msg.d); } }); }); </script>
Here in the above code you have seen that I have written code in document ready function of jQuery which will called when page will be loaded and with jQuery Ajax function I have called page method and replace the text of div with the reply from Page method. Now when you run this in browser. It’s look like following.
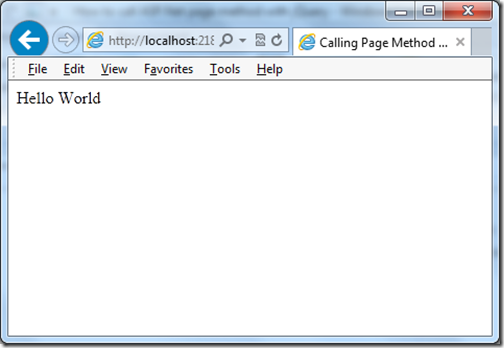
You can see It’s a very easy to call a page method in jQuery. Hope you like it. Stay tuned for more..
Technically you are calling a web method and not a page method. Am more interested in seeing how you call ASPX Page method and not WebMethod.
ReplyDeleteSanket - First of all asp.net web services and asp.net page methods are different. If you don't know the difference here is the link -
ReplyDeletehttp://stackoverflow.com/questions/6604050/differences-between-doing-ajax-using-a-page-method-a-web-service-and-a-custom-h
For web service you need different file .asmx and page method you don't need to create separate file. It's an asp.net ajax functionality and Microsoft it self uses term as page method.
Yes, It's similar to web services web method attribute. Because here also we have web method attribute. But that does not mean it's a web service.
And There is no way to directly call a server side method. Either you have to use page method or you need to implement call back interface.
Please see my wordings clearly - I said you are calling WebMethod. I never said as a Web Service. Never mind - mistakes do happen.
ReplyDeleteSecondly, your method is static.Is there a way I can differentiate between sessions across users?
Sanket, I have not made mistake.You are leading this conversation in wrong direction.I think you are mixing terms and getting confused. This asp.net page methods are there from ASP.NET 2.0. This is provides by default with script manager. The methods you are talking about is called "Server side methods" which we are write in .cs file. It is not called Pagemethods.
ReplyDeleteThis is same like comparing WCF with Windows Azure where both are separate entities.
There is no direct way to call server side method from javascript. There are workaround like creating static method and writing server brackets like <%=YourMethodName()%> . But it does not work on all the scenario and many drawbacks.
Regarding session, Yes, You can use session in page methods. See the this link- http://stackoverflow.com/questions/5554718/set-session-in-pagemethod-asp-net
But If you are calling multiple page method same time asyc way then you can not modify session it should be in read only-http://stackoverflow.com/questions/4506273/make-session-readonly-on-asp-net-programmatically-enablesessionstate-readonly
If you not make this session read only then it will call one by one.
I hope my answers will satisfy your query.
Regards,
Jalpesh
Its your call. It seems you are hurt by the statement. I've not mixed the terms - that's what I know.
ReplyDeletehttp://stackoverflow.com/questions/4264039/page-method-vs-web-service
ReplyDeletehttp://forums.asp.net/t/1381378.aspx/1
Those are my clear readings...Those articles give clear answer to my question.
Anyways, thanks for your attempt. :)
Well I never hurt by any comments. It's my duty to answer comments !!
ReplyDeleteThat's good you got your answer!!
ReplyDeleteThis comment has been removed by a blog administrator.
ReplyDeleteThere was a time when sharing knowledge was considered a noble contribution... but alas! nowadays it is just becoming a platform to display or hurt egos
ReplyDeleteguys, play a fair game :P