Recently I was looking into someone’s code found that they are using the throw(ex) to log exception so I told that person that why you are not using only throw he said there is no difference.. Wait this is not true there is a difference. So, this post is all about throw Vs. throw(ex) best practice and what is difference.
We all know that C# provides facility to handle exception with try and catch block and some times we use throw statement in catch block to throw the exception to log the exception. Here there two options either you could use throw(ex) or simple throw just like following.
And
So which one is good and best practice let’s study that.
Here in the above code I have created function DevideByZero where it will create a problem as it try to devide a integer by zero. and in try catch block I am use throw to again throw exception. Now let’s run this application.

Now I have just replace throw with throw(exception) and see it will have following output.
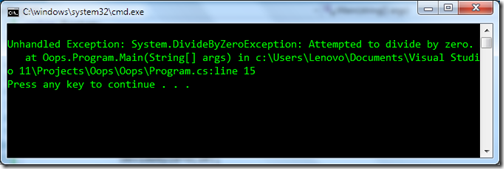
So you can see in first image we have got full stack trace information where the actual exception called at line 22 and again rethrow at line 15 While in the another screenshot you can see there is only information about line 15. It does not show full stack trace.So when you use throw(ex) it will reset stack trace.
So it’s always best practice to use throw instead of throw(ex). That’s it. Hope you like it..Stay tuned for more
We all know that C# provides facility to handle exception with try and catch block and some times we use throw statement in catch block to throw the exception to log the exception. Here there two options either you could use throw(ex) or simple throw just like following.
try { } catch (Exception ex) { throw; }
And
try { } catch (Exception ex) { throw(ex); }
So which one is good and best practice let’s study that.
Similarities:
Let’s first see what is similar in both.- Both are used to throw exception in catch block to log message
- Both contains same message of exception
Difference:
Now let’s see what is difference.- throw is used to throw current exception while throw(ex) mostly used to create a wrapper of exception.
- throw(ex) will reset your stack trace so error will appear from the line where throw(ex) written while throw does not reset stack trace and you will get information about original exception.
- In MSIL code when you use throw(ex) it will generate code as throw and if you use throw it will create rethrow.
using System; namespace Oops { class Program { static void Main(string[] args) { try { DevideByZero(10); } catch (Exception exception) { throw; } } public static void DevideByZero(int i) { int j = 0; int k = i/j; Console.WriteLine(k); } } }
Here in the above code I have created function DevideByZero where it will create a problem as it try to devide a integer by zero. and in try catch block I am use throw to again throw exception. Now let’s run this application.

Now I have just replace throw with throw(exception) and see it will have following output.
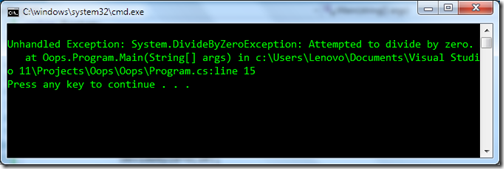
So you can see in first image we have got full stack trace information where the actual exception called at line 22 and again rethrow at line 15 While in the another screenshot you can see there is only information about line 15. It does not show full stack trace.So when you use throw(ex) it will reset stack trace.
So it’s always best practice to use throw instead of throw(ex). That’s it. Hope you like it..Stay tuned for more
