Before C# 4.0 there was no on demand initialization by default. So at the time of declaration we need to create a value or object will be null or we have to create on demand initialization via coding.. But with C# 4.0 we now have lazy class. As per MSDN it support lazy initialization so it means if we use lazy class then it will initialize the object at the time of demand.
It is very useful for UI responsiveness and other scenario's. Lazy initialization delays certain initialization and it’s improve the start-up time of a application. In the earlier framework if we need this kind of functionality we have to do it manually via coding but from C# 4.0 onwards it have Lazy<T> class. With the Help of this we can improve the responsiveness of C# application. Following is a simple example.
As you can see in the above example, I have created a class called Customer with public constructor and in constructor it prints that Customer class is initialized and that constructor will be called. In the main method of console application I have created lazy customer object with the help of lazy in c#. I have used IsValueCreated property to check whether value initialized or not and I am printing that values before the use and after use of lazy object.
Let’s run example via pressing F5 and following is a output as expected.
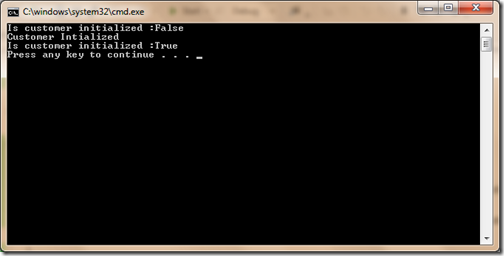
You can see in above example first value was not initialize so IsValueCreated returned false. After use of customer its initialized and its returns true.
Note: C# lazy initialization is complex process internally so it will have a overhead if you define all the object as lazy, So whenever the object could take time to initialize at that time you can use Lazy in c#. For example reading a file will take more time due to IO operations as well if you are fetching data from the third party source or web service where it can take longer time to initialize then you can use lazy over there.
That’s it. Hope you like it. Stay tuned for more..
It is very useful for UI responsiveness and other scenario's. Lazy initialization delays certain initialization and it’s improve the start-up time of a application. In the earlier framework if we need this kind of functionality we have to do it manually via coding but from C# 4.0 onwards it have Lazy<T> class. With the Help of this we can improve the responsiveness of C# application. Following is a simple example.
using System; namespace LazyExample { class Program { static void Main(string[] args) { Lazy<Customer> lazyCustomer = new Lazy<Customer>(); Console.WriteLine(string.Format("Is customer initialized :{0}", lazyCustomer.IsValueCreated)); Customer customer = lazyCustomer.Value; Console.WriteLine(string.Format("Is string value initialized :{0}", lazyCustomer.IsValueCreated)); } } public class Customer { public Customer() { Console.WriteLine("Customer Intialized"); } } }
As you can see in the above example, I have created a class called Customer with public constructor and in constructor it prints that Customer class is initialized and that constructor will be called. In the main method of console application I have created lazy customer object with the help of lazy in c#. I have used IsValueCreated property to check whether value initialized or not and I am printing that values before the use and after use of lazy object.
Let’s run example via pressing F5 and following is a output as expected.
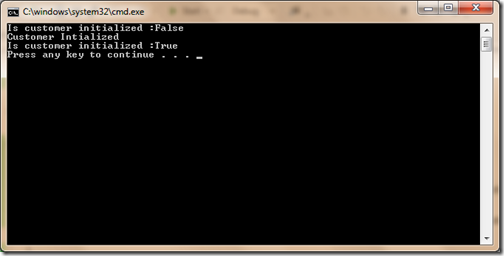
You can see in above example first value was not initialize so IsValueCreated returned false. After use of customer its initialized and its returns true.
Note: C# lazy initialization is complex process internally so it will have a overhead if you define all the object as lazy, So whenever the object could take time to initialize at that time you can use Lazy in c#. For example reading a file will take more time due to IO operations as well if you are fetching data from the third party source or web service where it can take longer time to initialize then you can use lazy over there.
That’s it. Hope you like it. Stay tuned for more..
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.