Recently some of colleagues were discussing about how to create overload methods in web service. We can’t directly create overloaded method web methods for web service. So I thought It would be great idea to write a blog post about it.
Function overloading is one of most known concepts of Object Oriented Programming. It’s a technique to implement polymorphism in code. Like in other programming language in c# you can also create multiple functions with same name and different argument. In function overloading function call will be resolved by ‘Best Match Technique’.
To understand this let’s create a simple web service with two HelloWorld Methods one with parameter and one without parameter both will return a string. Following is a code for that.
Now once you compile this code and run this application. You will get below error.
Here in the above code you can see that it is asking for message name property of web method so we need to create unique method name attributes and another thing is WsiProfiles.BasicProfile1_1 does not support method overloading so we have to make it none. Following is a code after modifications.
Now once you run web service. It will work like following
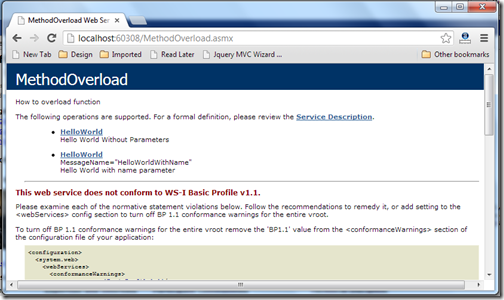
The reason for above error is because when WSDL generated for this web service. It will not make any difference between this two methods. So message name property of web method uniquely indentifies that. So it’s very easy to create method overloading in asp.net but You also know that it not supported in WSI standards(We have made None). That’s it. Hope you like it. Stay tuned for more.
Function Overloading:
Problem: You cannot directly overload method in web service:
using System.Web.Services; namespace WebApplication3 { [WebService(Namespace = "http://tempuri.org/", Description = "How to overload function")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] [System.ComponentModel.ToolboxItem(false)] public class MethodOverload : System.Web.Services.WebService { [WebMethod(MessageName = "HelloWorld", Description = "Hello World Without Parameters")] public string HelloWorld() { return "Hello World"; } [WebMethod(MessageName = "HelloWorldWithName", Description = "Hello World with name parameter")] public string HelloWorld(string name) { return string.Format("Hello Word {0}", name); } } }
Now once you compile this code and run this application. You will get below error.
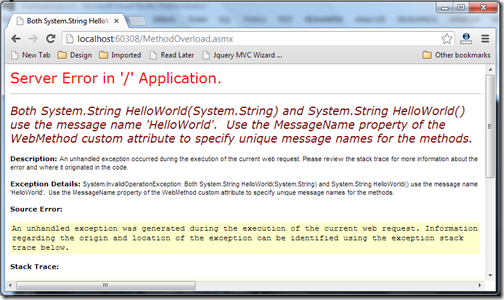
Solution: Function overloading in asp.net web service:
using System.Web.Services; namespace WebApplication3 { [WebService(Namespace = "http://tempuri.org/", Description = "How to overload function")] [WebServiceBinding(ConformsTo = WsiProfiles.None)] [System.ComponentModel.ToolboxItem(false)] public class MethodOverload : System.Web.Services.WebService { [WebMethod(MessageName = "HelloWorld", Description = "Hello World Without Parameters")] public string HelloWorld() { return "Hello World"; } [WebMethod(MessageName = "HelloWorldWithName", Description = "Hello World with name parameter")] public string HelloWorld(string name) { return string.Format("Hello Word {0}", name); } } }
Now once you run web service. It will work like following
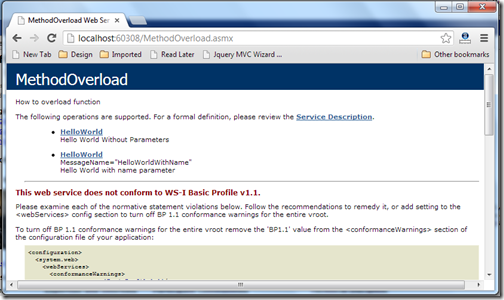
The reason for above error is because when WSDL generated for this web service. It will not make any difference between this two methods. So message name property of web method uniquely indentifies that. So it’s very easy to create method overloading in asp.net but You also know that it not supported in WSI standards(We have made None). That’s it. Hope you like it. Stay tuned for more.
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.