public void AddRange( IEnumerable<T> collection )To Understand let’s take simple example like following.
using System.Collections.Generic; using System; namespace Linq { class Program { static void Main(string[] args) { List<string> names=new List<string> {"Jalpesh"}; string[] newnames=new string[]{"Vishal","Tushar","Vikas","Himanshu"}; foreach (var newname in newnames) { names.Add(newname); } foreach (var n in names) { Console.WriteLine(n); } } } }
Here in the above code I am adding content of array to a already created list via foreach loop. You can use AddRange method instead of for loop like following.It will same output as above.
using System; using System.Collections.Generic; namespace Linq { class Program { static void Main(string[] args) { List<string> names=new List<string> {"Jalpesh"}; string[] newnames=new string[]{"Vishal","Tushar","Vikas","Himanshu"}; names.AddRange(newnames); foreach (var n in names) { Console.WriteLine(n); } } } }
Now when you run that example output is like following.
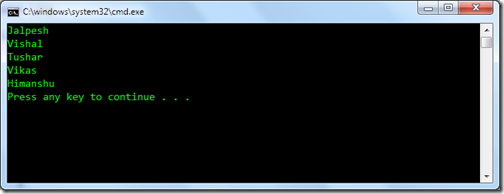
Add Range in more complex scenario:
You can also use add range to more complex scenarios also like following.You can use other operator with add range as following.using System; using System.Collections.Generic; using System.Linq; namespace Linq { class Program { static void Main(string[] args) { List<string> names=new List<string> {"Jalpesh"}; string[] newnames=new string[]{"Vishal","Tushar","Vikas","Himanshu"}; names.AddRange(newnames.Where(nn=>nn.StartsWith("Vi"))); foreach (var n in names) { Console.WriteLine(n); } } } }
Here in the above code I have created array with string and filter it with where operator while adding it to an existing list. Following is output as expected.
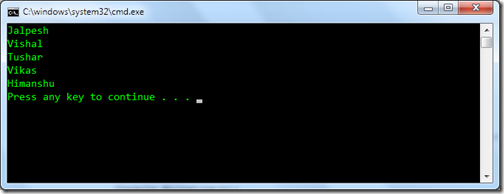
That’s it. Hope you like it. Stay tuned for more..
Awesome.
ReplyDeleteThanks
Delete