Before some time I have written a post about Getting Started with Nhibernate and ASP.NET MVC –CRUD operations. It’s one of the most popular post blog post on my blog. I get lots of questions via email and other medium why you are not writing a post about Fluent Nhibernate and ASP.NET MVC. So I thought it will be a good idea to write a blog post about it.
Convection over configuration that is mantra of Fluent Hibernate If you have see the my blog post about Nhibernate then you might have found that we need to create xml mapping to map table. Fluent Nhibernate uses POCO mapping instead of XML mapping and I firmly believe in Convection over configuration that is why I like Fluent Nhibernate a lot. But it’s a matter of Personal Test and there is no strong argument why you should use Fluent Nhibernate instead of Nhibernate.
Fluent Nhibernate is team Comprises of James Gregory, Paul Batum, Andrew Stewart and Hudson Akridge. There are lots of committers and it’s a open source project.
You can find all more information about at following site.
http://www.fluentnhibernate.org/
On this site you can find definition of Fluent Nhibernate like below.
https://github.com/jagregory/fluent-nhibernate/wiki/Getting-started
So all set it’s time to write a sample application. So from visual studio 2013 go to file – New Project and add a new web application project with ASP.NET MVC.
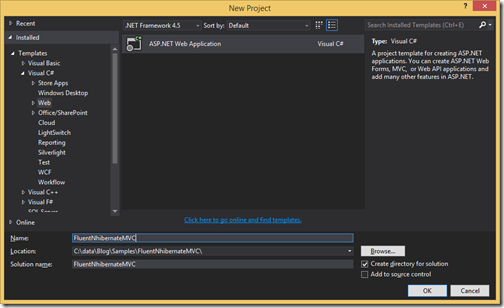
Once you are done with creating a web project with ASP.NET MVC It’s time to add Fluent Nhibernate to Application. You can add Fluent Nhibernate to application via following NuGet Package.

And you can install it with package manager console like following.
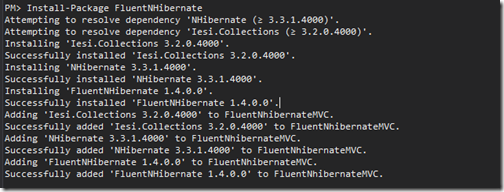
Now we are done with adding a Fluent Nhibernate to it’s time to add a new database.
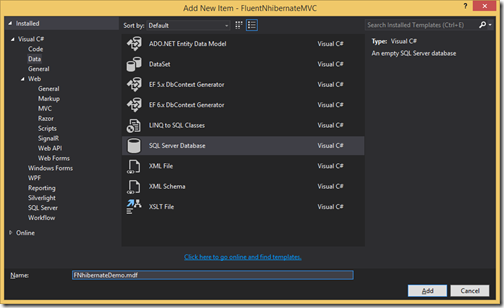
Now I’m going to create a simple table called “Employee” with four columns Id, FirstName, LastName and Designation. Following is a script for that.
Now Once we are done with our model classes and other stuff now it’s time to create a Map class which map our model class to our database table as we know that Fluent Nhibernate is using POCO classes to map instead of xml mappings. Following is a map class for that.
If you see Employee Map class carefully you will see that I have mapped Id column with EmployeeId in table and another things you can see I have written a table with “Employee” which will map Employee class to employee class.
Now once we are done with our mapping class now it’s time to add Nhibernate Helper which will connect to SQL Server database.
Now we are done with classes It’s time to scaffold our Controller for the application. Right click Controller folder and click on add new controller. It will ask for scaffolding items like following.
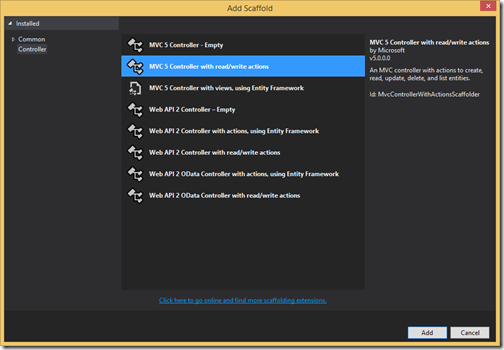
I have selected MVC5 controller with read/write action. Once you click Add It will ask for controller name.
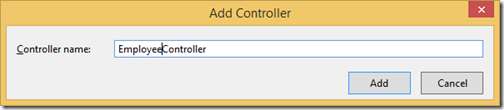
Now our controller is ready. It’s time to write code for our actions.
Listing:
Following is a code for our listing action.
You add view for listing via right click on View and Add View.
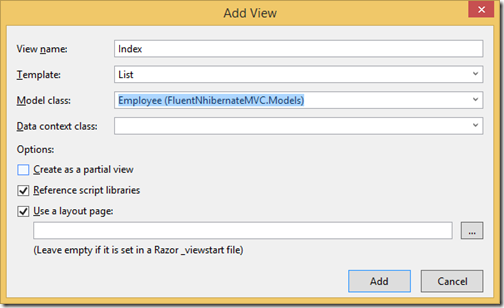
That’s is you are done with listing of your database. I have added few records to database table to see whether its working or not. Following is a output as expected.
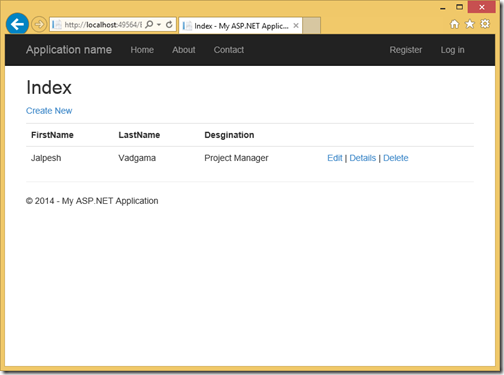
Create:
Now it’s time to create code and views for creating a Employee. Following is a code for action results.
Here you can see one if for blank action and another for posting data and saving to SQL Server with HttpPost Attribute. You can add view for create via right click on view on action result like following.
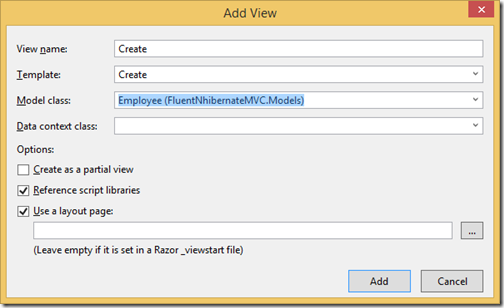
Now when you run this you will get output as expected.
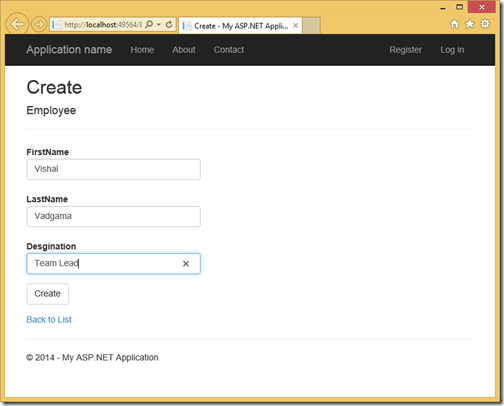
Once you click on create it will return back to listing screen like this.
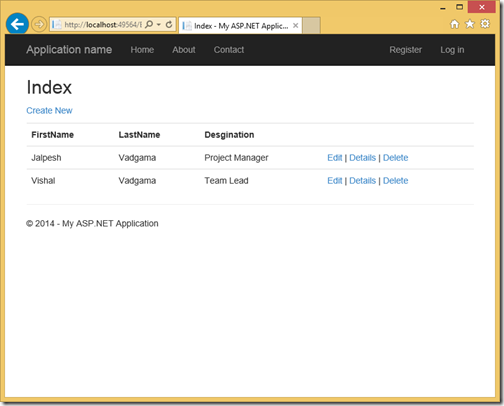
Edit:
Now we are done with listing/adding employee it’s time to write code for editing/updating employee. Following is a code Action Methods.
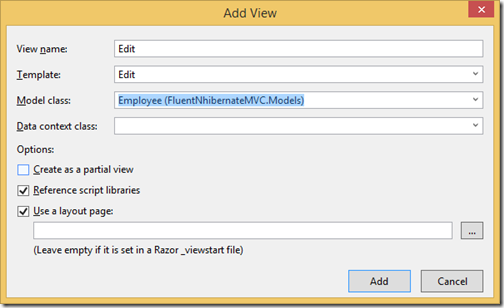
Now when you run application it will work as expected.
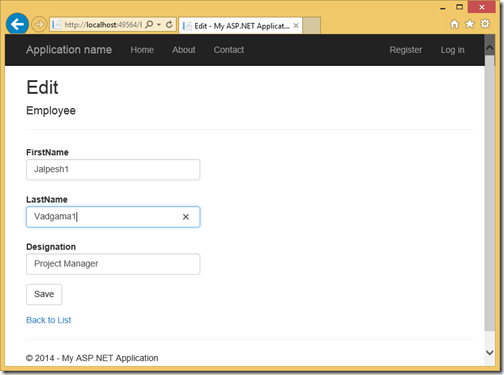
Details:
You can write details code like following.
You can view via right clicking on view as following.
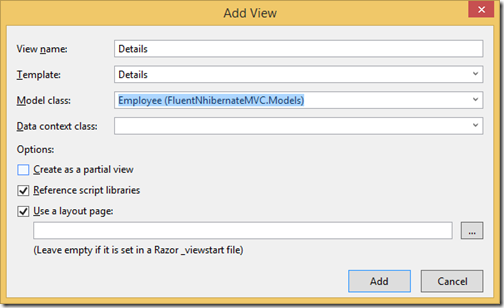
Now when you run application following is a output as expected.
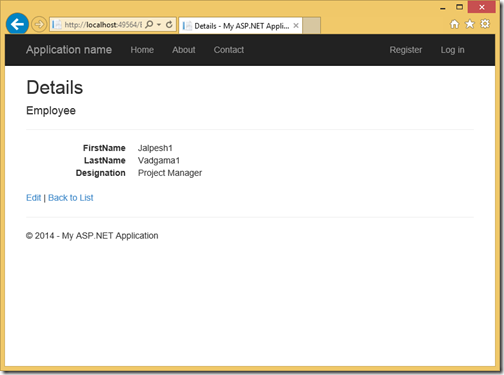
Delete:
Following is a code for deleting Employee one action method for confirmation of delete and another one for deleting data from employee table.
You can add view for delete via right click on view like following.
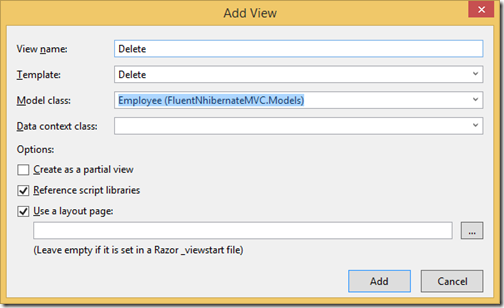
When you run it’s running as expected.
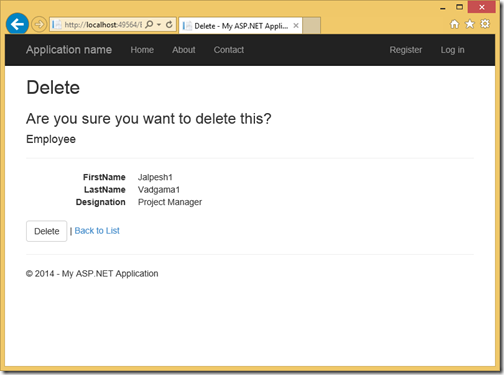
Once you click on delete it will delete employee. That’s it. You can see it’s very easy. Fluent Nhibernate supports POCO mapping without writing any complex xml mappings.
You can find whole source code at GitHub at following location.
https://github.com/dotnetjalps/FluentNhiberNateMVC
Hope you like it. Stay tuned for more!!
What is Fluent Nhibernate:
Fluent Nhibernate is team Comprises of James Gregory, Paul Batum, Andrew Stewart and Hudson Akridge. There are lots of committers and it’s a open source project.
You can find all more information about at following site.
http://www.fluentnhibernate.org/
On this site you can find definition of Fluent Nhibernate like below.
Fluent, XML-less, compile safe, automated, convention-based mappings for NHibernate. Get your fluent on.They have excellent getting started guide on following url. You can easily walk through it and learned it.
https://github.com/jagregory/fluent-nhibernate/wiki/Getting-started
ASP.NET MVC and Fluent Nhibernate:
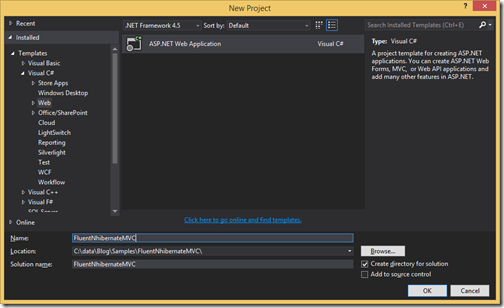
Once you are done with creating a web project with ASP.NET MVC It’s time to add Fluent Nhibernate to Application. You can add Fluent Nhibernate to application via following NuGet Package.

And you can install it with package manager console like following.
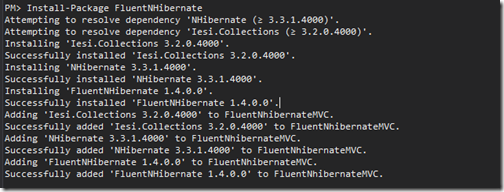
Now we are done with adding a Fluent Nhibernate to it’s time to add a new database.
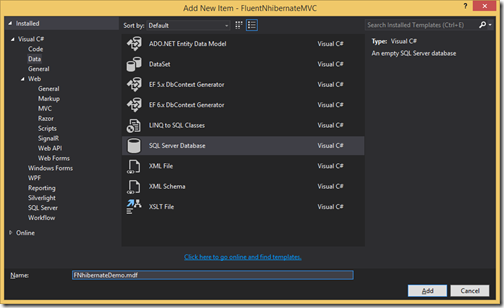
Now I’m going to create a simple table called “Employee” with four columns Id, FirstName, LastName and Designation. Following is a script for that.
CREATE TABLE [dbo].[Employee] ( [Id] INT NOT NULL PRIMARY KEY, [FirstName] NVARCHAR(50) NULL, [LastName] NVARCHAR(50) NULL, [Designation] NVARCHAR(50) NULL )Now table is ready it’s time to create a Model class for Employee. So following is a code for that.
namespace FluentNhibernateMVC.Models { public class Employee { public virtual int EmployeeId { get; set; } public virtual string FirstName { get; set; } public virtual string LastName { get; set; } public virtual string Designation{ get; set; } } }
Now Once we are done with our model classes and other stuff now it’s time to create a Map class which map our model class to our database table as we know that Fluent Nhibernate is using POCO classes to map instead of xml mappings. Following is a map class for that.
using FluentNHibernate.Mapping; namespace FluentNhibernateMVC.Models { public class EmployeeMap : ClassMap<Employee> { public EmployeeMap() { Id(x => x.EmployeeId); Map(x => x.FirstName); Map(x => x.LastName); Map(x => x.Designation); Table("Employee"); } } }
If you see Employee Map class carefully you will see that I have mapped Id column with EmployeeId in table and another things you can see I have written a table with “Employee” which will map Employee class to employee class.
Now once we are done with our mapping class now it’s time to add Nhibernate Helper which will connect to SQL Server database.
using FluentNHibernate.Cfg; using FluentNHibernate.Cfg.Db; using NHibernate; using NHibernate.Tool.hbm2ddl; namespace FluentNhibernateMVC.Models { public class NHibernateHelper { public static ISession OpenSession() { ISessionFactory sessionFactory = Fluently.Configure() .Database(MsSqlConfiguration.MsSql2008 .ConnectionString(@"Data Source=(LocalDB)\v11.0;AttachDbFilename=C:\data\Blog\Samples\FluentNhibernateMVC\FluentNhibernateMVC\FluentNhibernateMVC\App_Data\FNhibernateDemo.mdf;Integrated Security=True") .ShowSql() ) .Mappings(m => m.FluentMappings .AddFromAssemblyOf<Employee>()) .ExposeConfiguration(cfg => new SchemaExport(cfg) .Create(false, false)) .BuildSessionFactory(); return sessionFactory.OpenSession(); } } }
Now we are done with classes It’s time to scaffold our Controller for the application. Right click Controller folder and click on add new controller. It will ask for scaffolding items like following.
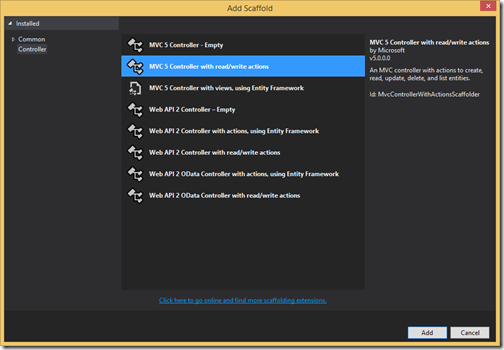
I have selected MVC5 controller with read/write action. Once you click Add It will ask for controller name.
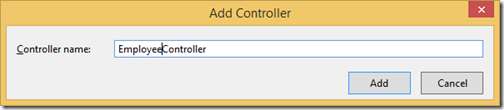
Now our controller is ready. It’s time to write code for our actions.
Listing:
Following is a code for our listing action.
public ActionResult Index() { using (ISession session = NHibernateHelper.OpenSession()) { var employees = session.Query<Employee>().ToList(); return View(employees); } }
You add view for listing via right click on View and Add View.
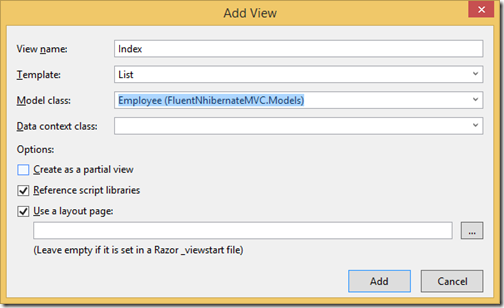
That’s is you are done with listing of your database. I have added few records to database table to see whether its working or not. Following is a output as expected.
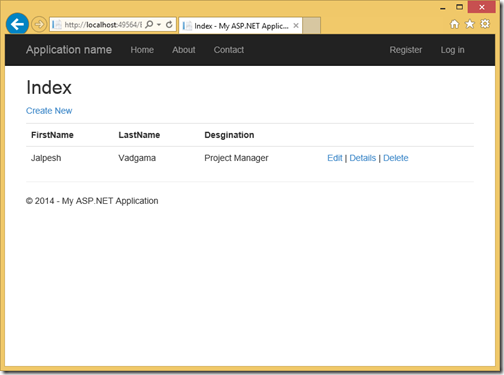
Create:
Now it’s time to create code and views for creating a Employee. Following is a code for action results.
public ActionResult Create() { return View(); } // POST: Employee/Create [HttpPost] public ActionResult Create(Employee employee) { try { using (ISession session = NHibernateHelper.OpenSession()) { using (ITransaction transaction = session.BeginTransaction()) { session.Save(employee); transaction.Commit(); } } return RedirectToAction("Index"); } catch (Exception exception) { return View(); } }
Here you can see one if for blank action and another for posting data and saving to SQL Server with HttpPost Attribute. You can add view for create via right click on view on action result like following.
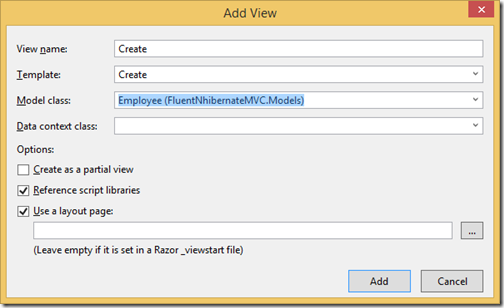
Now when you run this you will get output as expected.
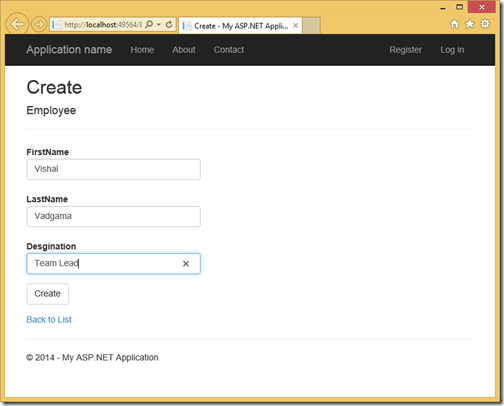
Once you click on create it will return back to listing screen like this.
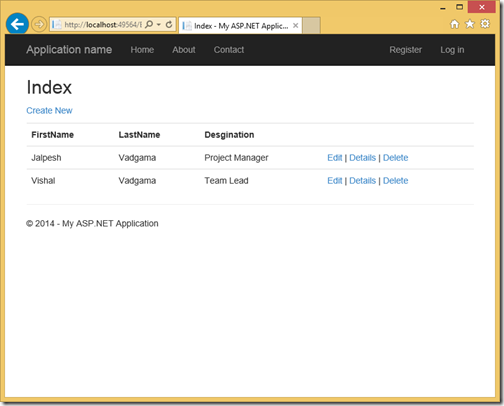
Edit:
Now we are done with listing/adding employee it’s time to write code for editing/updating employee. Following is a code Action Methods.
public ActionResult Edit(int id) { using (ISession session = NHibernateHelper.OpenSession()) { var employee = session.Get<Employee>(id); return View(employee); } } // POST: Employee/Edit/5 [HttpPost] public ActionResult Edit(int id, Employee employee) { try { using (ISession session = NHibernateHelper.OpenSession()) { var employeetoUpdate = session.Get<Employee>(id); employeetoUpdate.Designation = employee.Designation; employeetoUpdate.FirstName = employee.FirstName; employeetoUpdate.LastName = employee.LastName; using (ITransaction transaction = session.BeginTransaction()) { session.Save(employeetoUpdate); transaction.Commit(); } } return RedirectToAction("Index"); } catch { return View(); } }You can create edit view via following via right click on view.
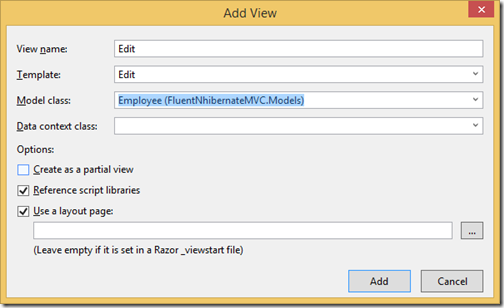
Now when you run application it will work as expected.
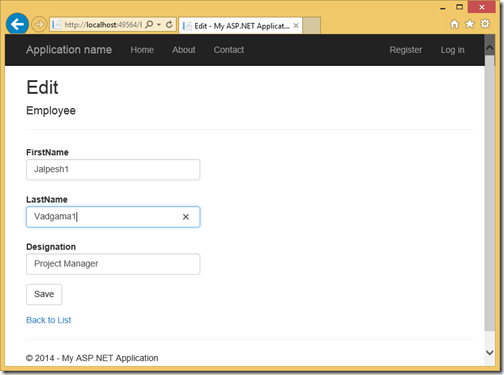
Details:
You can write details code like following.
public ActionResult Details(int id) { using (ISession session = NHibernateHelper.OpenSession()) { var employee = session.Get<Employee>(id); return View(employee); } }
You can view via right clicking on view as following.
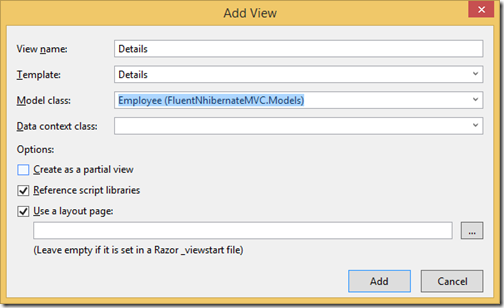
Now when you run application following is a output as expected.
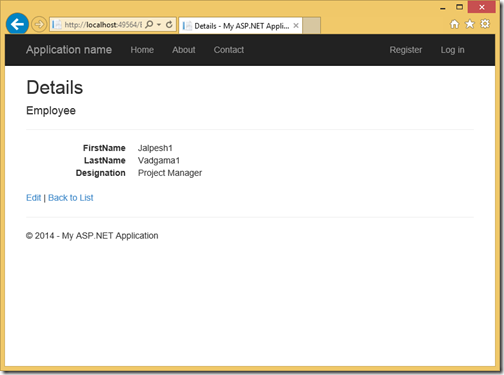
Delete:
Following is a code for deleting Employee one action method for confirmation of delete and another one for deleting data from employee table.
public ActionResult Delete(int id) { using (ISession session = NHibernateHelper.OpenSession()) { var employee = session.Get<Employee>(id); return View(employee); } } [HttpPost] public ActionResult Delete(int id, Employee employee) { try { using (ISession session = NHibernateHelper.OpenSession()) { using (ITransaction transaction = session.BeginTransaction()) { session.Delete(employee); transaction.Commit(); } } return RedirectToAction("Index"); } catch (Exception exception) { return View(); } }
You can add view for delete via right click on view like following.
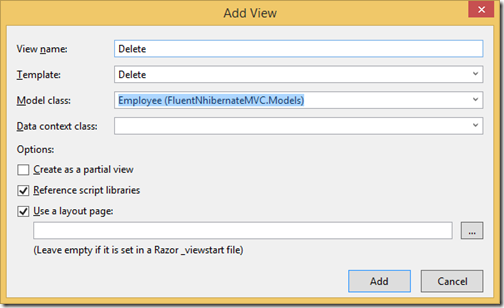
When you run it’s running as expected.
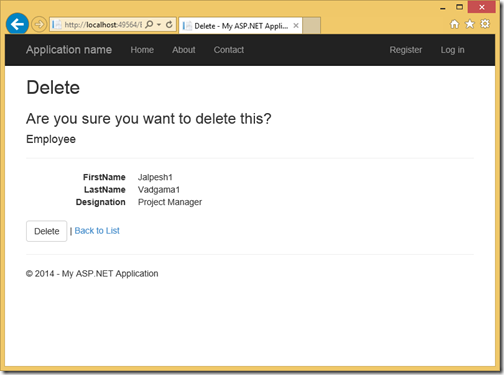
Once you click on delete it will delete employee. That’s it. You can see it’s very easy. Fluent Nhibernate supports POCO mapping without writing any complex xml mappings.
You can find whole source code at GitHub at following location.
https://github.com/dotnetjalps/FluentNhiberNateMVC
Hope you like it. Stay tuned for more!!
Very useful and easy to understand for those who are new to MVC. Thank you for sharing this article. Really a great work.
ReplyDeleteYou're welcome and thanks for visiting my blog
DeleteThanks!
ReplyDeleteYou're welcome
Delete