In this post we are going to learn about How we can do dependency injection with StructureMap. Here we are going to take a sample application of shopping cart. This shopping cart can process two type of orders 1. Sales order 2. Purchase Order. We want an abstraction for this. So first we are going to create an interface IOrder which will be implemented by both Purchase Order and Sales Order classes.
Following is a code for that.
Now If you see my shopping cart implementation I have created a readonly object of IOrder which I initialized with Constructor of Shopping Cart.
Now let’s write some code Main method to do checkout process for shopping cart. Following is a code for that.
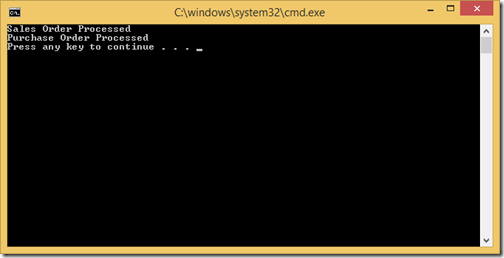
Now if you see the implementation of main method code carefully then you can see I have created two object for Sales Order and Purchase Order. This where an IOC(Inversion of Control) container can help we can give responsibilities of object creation to IOC container. Here we are going to utilize StructureMap as IOC container.
StructureMap is one of the oldest IOC Container which was started in 2004. It’s a open source project and available under Apache licence. It was primary started by Jeremy Miller and then lot of developers contributed to it.
You can get more information about it in following link.
http://structuremap.github.io/
Now it’s time to add StructureMap to our project. You can add it via NuGet Package following is a command for StructureMap.

Once you are done with Installing Nuget Pacakge. It’s time to write some code for the same. I have changed my main method code like following.
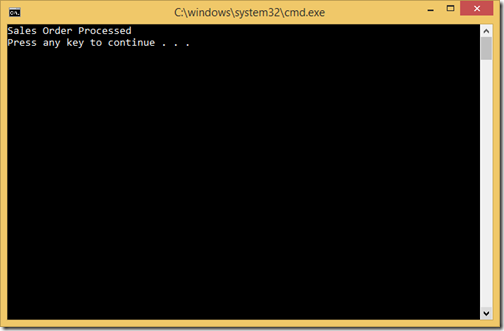
So you can see we have created an Dependency Injection with StructureMap. I have purposely not created object of Purchase Order. It will be a topic of our next post related to StructureMap. Stay tuned!!
Following is a code for that.
public interface IOrder { void Process(); }And following is a implementation of SalesOrder class.
public class SalesOrder : IOrder { public void Process() { Console.WriteLine("Sales Order Processed"); } }Same way following is a implementation of PurchaseOrder class.
public class PurchaseOrder : IOrder { public void Process() { Console.WriteLine("Purchase Order Processed"); } }And following is a code for Shopping Cart.
public class ShoppingCart { private readonly IOrder _order; public ShoppingCart(IOrder order) { _order = order; } public void CheckOut() { _order.Process(); } }
Now If you see my shopping cart implementation I have created a readonly object of IOrder which I initialized with Constructor of Shopping Cart.
Now let’s write some code Main method to do checkout process for shopping cart. Following is a code for that.
namespace StructureMapIOC { class Program { static void Main(string[] args) { IOrder saleOrder=new SalesOrder(); ShoppingCart salesShoppingCart=new ShoppingCart(saleOrder); salesShoppingCart.CheckOut(); IOrder purchaseoOrder=new PurchaseOrder(); ShoppingCart purchaseShoppingCart=new ShoppingCart(purchaseoOrder); purchaseShoppingCart.CheckOut(); } } }Now let's run application and it will output as following.
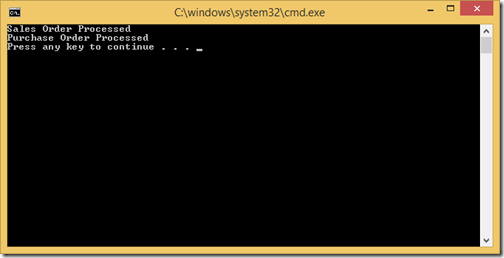
Now if you see the implementation of main method code carefully then you can see I have created two object for Sales Order and Purchase Order. This where an IOC(Inversion of Control) container can help we can give responsibilities of object creation to IOC container. Here we are going to utilize StructureMap as IOC container.
About StructureMap:
You can get more information about it in following link.
http://structuremap.github.io/
Using StructureMap:

Once you are done with Installing Nuget Pacakge. It’s time to write some code for the same. I have changed my main method code like following.
using StructureMap; namespace StructureMapIOC { class Program { static void Main(string[] args) { var container = new Container(); container.Configure(x=>x.For<IOrder>().Use<SalesOrder>()); ShoppingCart shoppingCart = container.GetInstance<ShoppingCart>(); shoppingCart.CheckOut(); } } }Here you can see that I have created container object of StructureMap and then I have configured the StructureMap to create a object of Sales Order Class and then you can see when I create object of ShoppingCart with GetInstance it will automatically create Sales Order Class Object. Now let’s run this application.
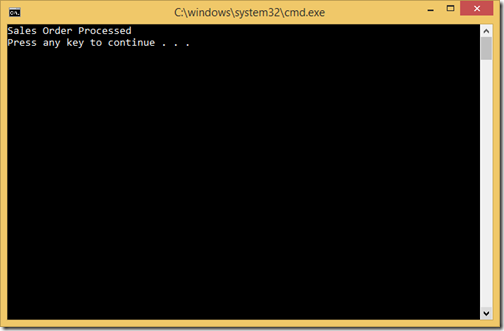
So you can see we have created an Dependency Injection with StructureMap. I have purposely not created object of Purchase Order. It will be a topic of our next post related to StructureMap. Stay tuned!!
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.