Now days, Convention over configured is more preferred and that’s why Fluent Nhibernate got more popularity Because with Fluent Nhibernate we don’t have to write and maintain mapping in xml. You can write classes to map your domain objects to your database tables. If you want to learn how we can do this with existing database and Fluent Nhibernate, I have also written a blog post about CRUD operation with Fluent Nhibernate and ASP.NET MVC . After writing this blog post I was getting a lots of emails about how we can create database from the Fluent Nhibernate as we can do same with Entity Framework Code First. So I thought it’s a good idea to write a blog post about it instead of writing individual emails.
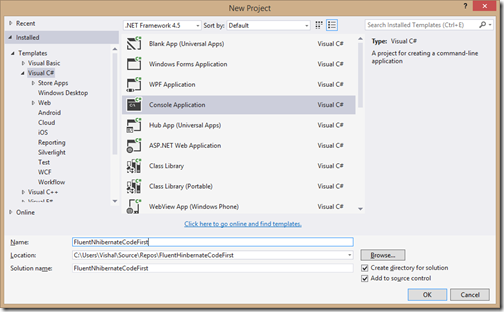
Once you are done with creating application. It’s time to add reference for Fluent Nhibernate. You can do this via your library package manager like following.
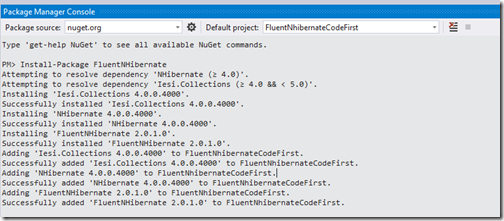
Now once, done with adding Fluent Nhibernate code, I have created a simple class “Customer” like below.
After creating table, I have initialize the customer object and saved it into database. Now when you run this application. You will get output like below as expected.
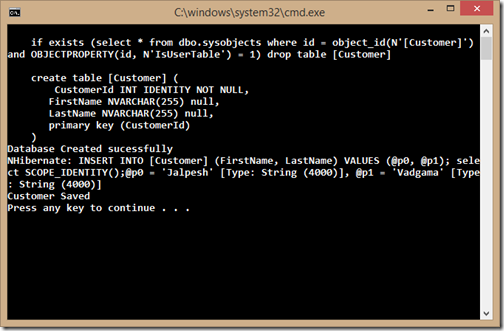
And now if you see database in the SQL Management Studio, Customer table has created like below.
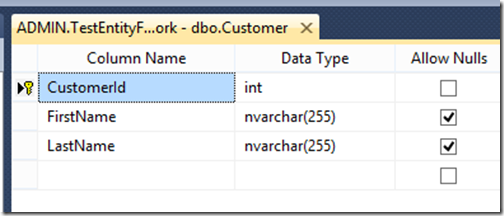
And If you see that table, Data is also inserted like below.
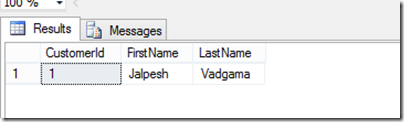
That’s it. Hope you like it. Stay tuned for more!.
How to create tables from class via Fluent Nhibernate:
To Demonstrate how we can create tables based mapping classes create we’re going to create a console application via adding new project like below.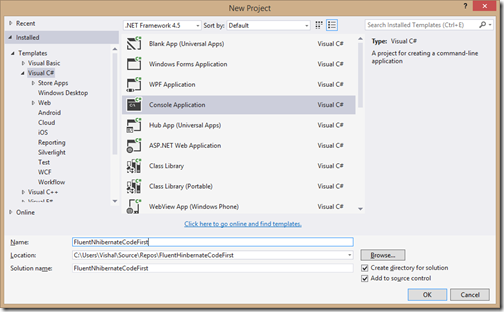
Once you are done with creating application. It’s time to add reference for Fluent Nhibernate. You can do this via your library package manager like following.
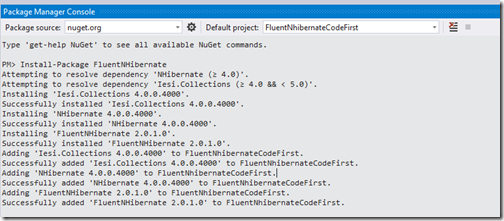
Now once, done with adding Fluent Nhibernate code, I have created a simple class “Customer” like below.
namespace FluentNhibernateCodeFirst { public class Customer { public virtual int CustomerId { get; set; } public virtual string FirstName { get; set; } public virtual string LastName { get; set; } } }Here you can see I have created three properties which will be also column of our database table. Now as we know we need to write a mapping class for customer so below is my customer map class.
using FluentNHibernate.Mapping; namespace FluentNhibernateCodeFirst { public class CustomerMap : ClassMap<Customer> { public CustomerMap() { Id(c => c.CustomerId); Map(c => c.FirstName); Map(c => c.LastName); } } }Here, I map ID Customer Id as customer Id will be primary key. Now it’s time to write code creating database and saving some data into customer table created.
using System; using System.Configuration; using FluentNHibernate.Cfg; using FluentNHibernate.Cfg.Db; using NHibernate; using NHibernate.Tool.hbm2ddl; namespace FluentNhibernateCodeFirst { class Program { private static ISessionFactory _sessionFactory; static void Main(string[] args) { //creating database string connectionString = ConfigurationManager.ConnectionStrings["DefaultConnectionString"].ConnectionString; CreateDatabase(connectionString); Console.WriteLine("Database Created sucessfully"); //creating a object of customer Customer customer=new Customer { CustomerId = 1, FirstName = "Jalpesh", LastName = "Vadgama" }; //saving customer in database. using(ISession session = _sessionFactory.OpenSession()) session.Save(customer); Console.WriteLine("Customer Saved"); } static void CreateDatabase(string connectionString) { var configuration = Fluently.Configure() .Database(MsSqlConfiguration.MsSql2012.ConnectionString(connectionString).ShowSql) .Mappings(m => m.FluentMappings.AddFromAssemblyOf<CustomerMap>()) .BuildConfiguration(); var exporter = new SchemaExport(configuration); exporter.Execute(true, true, false); _sessionFactory = configuration.BuildSessionFactory(); } } }Here in the above code, If you above code care fully then, I have created function called CreateDatabase. In this function First it will create a mapping and then that schema mapping will be executed against database to create table.
After creating table, I have initialize the customer object and saved it into database. Now when you run this application. You will get output like below as expected.
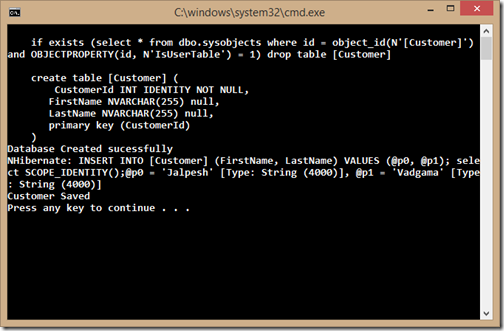
And now if you see database in the SQL Management Studio, Customer table has created like below.
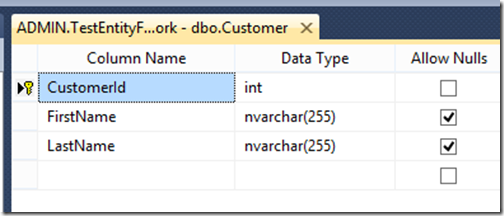
And If you see that table, Data is also inserted like below.
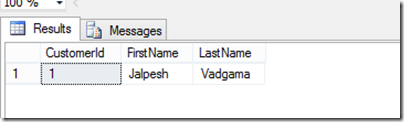
That’s it. Hope you like it. Stay tuned for more!.
You can find complete sourcecode of this example at github on -https://github.com/dotnetjalps/FluentHinbernateCodeFirst
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.