There are lots of ways to implement repository pattern but with ado.net entity model you can create repository pattern within 10 to 15 minutes.
If you are creating asp.net mvc application then just right click->Model folder->Add->Net Item->select ado.net entity data model.
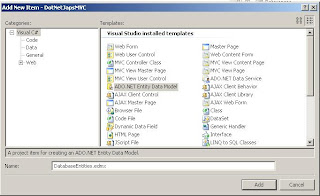
From database explorer right click and drag your tables to your database explorer. It will create a entity class. For example i have created a notes table for my sample and it will create a notes entity class in my ado.net entity data model.
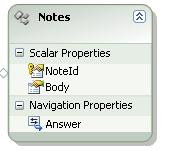
After creating the entity class not its time to create a interface for repository pattern which will contain my all the operations related to notes entity.
Following is the interface which we will implement use for repository.
namespace DotNetJapsMVC.Models.Respository.Interface
{
interface INotesRepository
{
void CreateNotes(Notes notesToCreate);
void EditNotes(Notes notesToEdit);
void DeleteNotes(Notes notesToDelete);
Notes GetNotes(Guid noteId);
IEnumerable ListNotes();
}
}
Now we will create a class that will implement this repository interface. Here is the coding for that.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace DotNetJapsMVC.Models.Respository.Class
{
public class NotesRepository:Interface.INotesRepository
{
private DotNetJapsEntities _myEntities = new DotNetJapsEntities();
public void CreateNotes(Notes notesToCreate)
{
_myEntities.AddToNotes(notesToCreate);
_myEntities.SaveChanges();
}
public void EditNotes(Notes notesToEdit)
{
var originalNotes = (from n in _myEntities.Notes
where n.NoteId == notesToEdit.NoteId
select n).FirstOrDefault();
_myEntities.ApplyPropertyChanges(originalNotes.EntityKey.EntitySetName, notesToEdit);
_myEntities.SaveChanges();
}
public void DeleteNotes(Notes notesToDelete)
{
var originalNotes = GetNotes(notesToDelete.NoteId);
_myEntities.DeleteObject(originalNotes );
_myEntities.SaveChanges();
}
public Notes GetNotes(Guid noteId)
{
return (from n in _myEntities.Notes
where n.NoteId == noteId
select n ).FirstOrDefault();
}
public IEnumerable ListNotes()
{
return _myEntities.Notes.ToList();
}
}
}
That's it we have created the repository classes and repository interface. You can use as following in your class or pages.
If you are creating asp.net mvc application then just right click->Model folder->Add->Net Item->select ado.net entity data model.
From database explorer right click and drag your tables to your database explorer. It will create a entity class. For example i have created a notes table for my sample and it will create a notes entity class in my ado.net entity data model.
After creating the entity class not its time to create a interface for repository pattern which will contain my all the operations related to notes entity.
Following is the interface which we will implement use for repository.
namespace DotNetJapsMVC.Models.Respository.Interface
{
interface INotesRepository
{
void CreateNotes(Notes notesToCreate);
void EditNotes(Notes notesToEdit);
void DeleteNotes(Notes notesToDelete);
Notes GetNotes(Guid noteId);
IEnumerable
}
}
Now we will create a class that will implement this repository interface. Here is the coding for that.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace DotNetJapsMVC.Models.Respository.Class
{
public class NotesRepository:Interface.INotesRepository
{
private DotNetJapsEntities _myEntities = new DotNetJapsEntities();
public void CreateNotes(Notes notesToCreate)
{
_myEntities.AddToNotes(notesToCreate);
_myEntities.SaveChanges();
}
public void EditNotes(Notes notesToEdit)
{
var originalNotes = (from n in _myEntities.Notes
where n.NoteId == notesToEdit.NoteId
select n).FirstOrDefault();
_myEntities.ApplyPropertyChanges(originalNotes.EntityKey.EntitySetName, notesToEdit);
_myEntities.SaveChanges();
}
public void DeleteNotes(Notes notesToDelete)
{
var originalNotes = GetNotes(notesToDelete.NoteId);
_myEntities.DeleteObject(originalNotes );
_myEntities.SaveChanges();
}
public Notes GetNotes(Guid noteId)
{
return (from n in _myEntities.Notes
where n.NoteId == noteId
select n ).FirstOrDefault();
}
public IEnumerable
{
return _myEntities.Notes.ToList();
}
}
}
That's it we have created the repository classes and repository interface. You can use as following in your class or pages.
private INotesRepository _repository; public ListLoadNotes() { return _repository.ListNotes(); } Advantage Of Repository Pattern:
- Make application loosely coupled so if you want to change the something then you don't need to change everything from scratch.
- With the repository pattern we can have nice abstraction which will separate our business logic as well as database logic.
- You can prevent dependency injection through the repository pattern.
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.