In this blog post, We are going to learn about Entity Framework feature. Explicit loading means that related data is explicitly loaded from the database at a later time. As you might know, that lazy loading is still not possible with Entity Framework core but there is a way to explicit load related data in a transparent manner. We are going explore how we can load data explicitly with entity framework in this blog post in detail.
So let’s create a console application like following.

Now once you click “Ok” it will create a console application. Now let’s add nuget package for entity framework core in console application like following. You need to run following command in Package Manager Console.

Now it’s time to create our models for Student and Department like below.
Department:
Now let’s create a migration to create the database for the same. To enable migration we need to install following nuget package for entity framework core tools.

Now let’s create a migration with the following command.

Now let’s create a database with “Update-database” in Package manager console. It will create a database.
Now we need some initial data to demonstrate the explicit loading feature so I’ve added following data into Departments table.

Same way I have added data into Students table like following.

Now it’s time to write some code that demonstrates the explicit loading feature of entity framework core. Following is a code for the same.
Now let’s run this application and here is the output as expected.
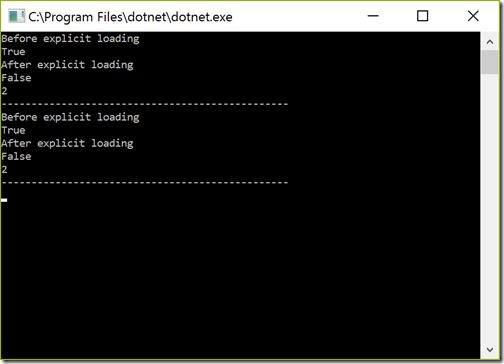
So it is loading student data after the department is loaded with the help of explicitly. That’s it. Hope you like it. There are so many scenarios where this explicit loading can be quite useful. Stay tuned for the more!!.
How to do Explicit loading in Entity Framework Core?
To demonstrate how we can use explicit loading in Entity Framework core. We are going to create a console application with two entities Student and Department. A department can have multiple students. Here we are going to see how we can load students for each department explicitly.So let’s create a console application like following.

Now once you click “Ok” it will create a console application. Now let’s add nuget package for entity framework core in console application like following. You need to run following command in Package Manager Console.
Install-Package Microsoft.EntityFrameworkCore.SqlServer

Now it’s time to create our models for Student and Department like below.
Department:
using System.Collections.Generic; namespace EFCoreExplicitLoading { public class Department { public int DepartmentId { get; set; } public string Name { get; set; } public ICollection<Student> Students { get; set; } } }Student:
namespace EFCoreExplicitLoading { public class Student { public int StudentId { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public int DepartMentId { get; set; } public Department Department { get; set; } } }Now let’s create an Entity Framework Core context like below.
using Microsoft.EntityFrameworkCore; namespace EFCoreExplicitLoading { public class StudentContext: DbContext { public DbSet<Student> Students { get; set; } public DbSet<Department> Departments { get; set; } protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { optionsBuilder.UseSqlServer(@"Data Source=SQLServerName;Initial Catalog=YourDatabase;User ID=UserName;Password=Password;MultipleActiveResultSets=true"); } } }Here in the above code, you see that A department can have multiple students and A student can have only one department so there is one to many relationships between department and student.
Now let’s create a migration to create the database for the same. To enable migration we need to install following nuget package for entity framework core tools.
Install-Package Microsoft.EntityFrameworkCore.Tools

Now let’s create a migration with the following command.
Add-Migration InitialDatabase

Now let’s create a database with “Update-database” in Package manager console. It will create a database.
Now we need some initial data to demonstrate the explicit loading feature so I’ve added following data into Departments table.

Same way I have added data into Students table like following.

Now it’s time to write some code that demonstrates the explicit loading feature of entity framework core. Following is a code for the same.
using System; using System.Linq; namespace EFCoreExplicitLoading { class Program { static void Main(string[] args) { using(StudentContext studentConext= new StudentContext()) { var deaprtments = studentConext.Departments.ToList(); foreach(var department in deaprtments) { Console.WriteLine("Before explicit loading"); Console.WriteLine(department.Students==null); //loading student explicitly studentConext.Entry(department).Collection(s => s.Students).Load(); Console.WriteLine("After explicit loading"); Console.WriteLine(department.Students == null); Console.WriteLine(department.Students.Count); Console.WriteLine("------------------------------------------------"); } Console.ReadLine(); } } } }Here in the above code, you can see I have created student context object and then I have got all the databases. After that, I have checked that whether each department is having students or not. In the next statement, I have loaded the students explicitly with the student with collection load method and then again I checking whether it got students and also printing count of a student.
Now let’s run this application and here is the output as expected.
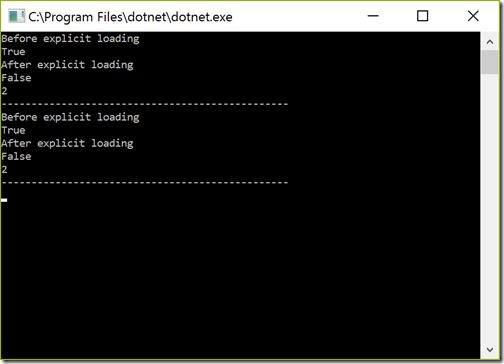
So it is loading student data after the department is loaded with the help of explicitly. That’s it. Hope you like it. There are so many scenarios where this explicit loading can be quite useful. Stay tuned for the more!!.
The complete source code of this sample application is available on github at - https://github.com/dotnetjalps/EFCodeExplicitLoading
0 comments:
Post a Comment
Your feedback is very important to me. Please provide your feedback via putting comments.